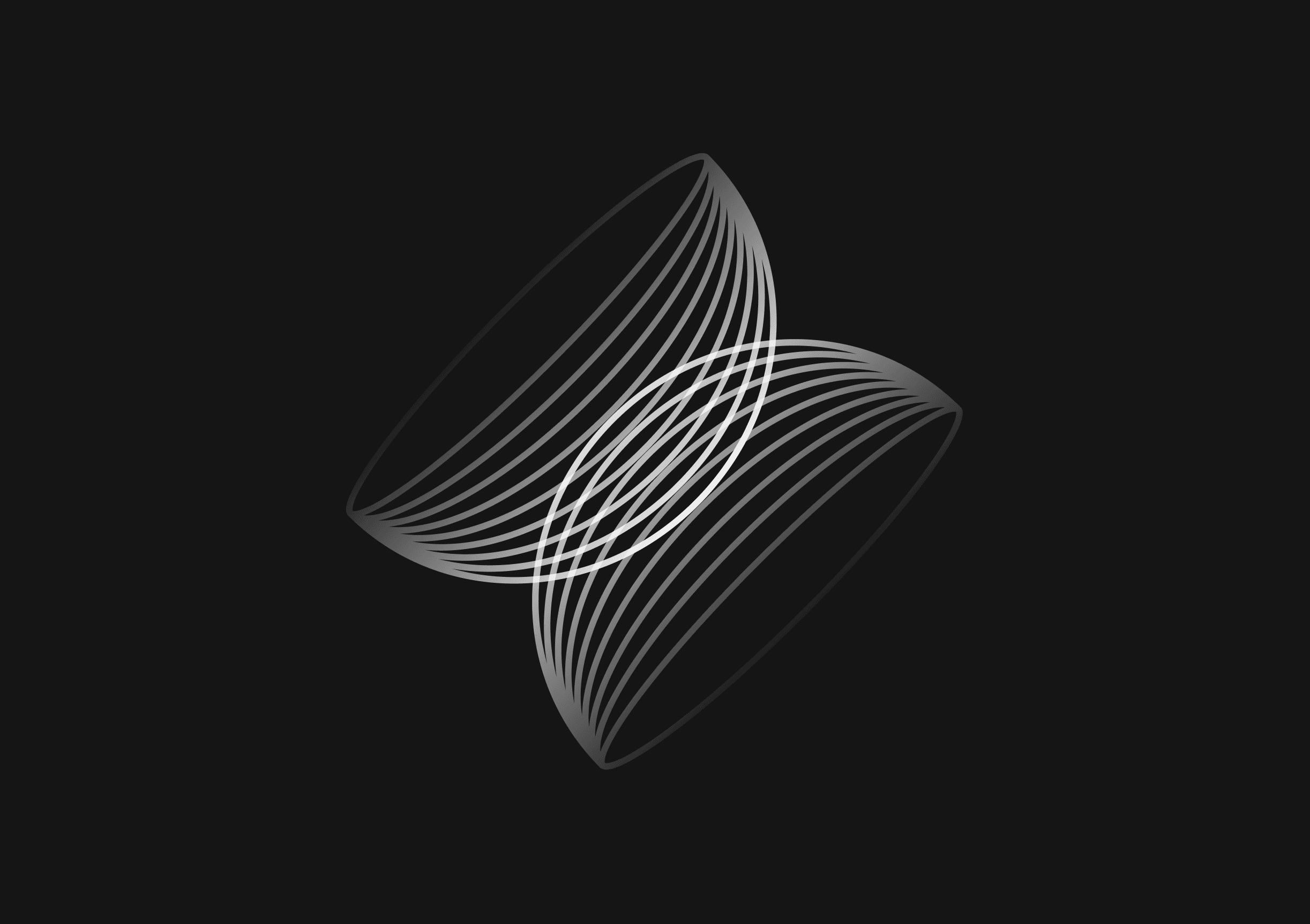
How to use a variable as a key in JavaScript
November 7, 2023
Using a variable as a key in JavaScript allows for dynamic assignment and access to properties within objects. It's a flexible way to handle property identifiers that are determined at runtime.
Understanding objects and keys in JavaScript
Objects in JavaScript are collections of properties, and properties are key-value pairs. A key (or property name) can be any string or symbol, while the value can be any valid JavaScript value.
let obj = { key: 'value' };
Defining a variable as a key
You can define a variable to use as a key using square brackets []
in an object literal. This is known as computed property names.
let keyName = 'dynamicKey'; let obj = { [keyName]: 'value' }; console.log(obj.dynamicKey); // outputs 'value'
Using variables for dynamic properties
When creating an object, if the property names are not known until runtime, you can use a variable as the key.
function createObject(key, value) { return { [key]: value }; } let obj = createObject('id', 1); console.log(obj.id); // outputs 1
Modifying object properties with variable keys
To modify the value of a property using a variable key, use the variable within square brackets to access the property.
let obj = { id: 1 }; let keyName = 'id'; obj[keyName] = 2; console.log(obj.id); // outputs 2
Accessing properties dynamically
You can also use a variable to access properties of an object dynamically.
let obj = { id: 1, name: 'JavaScript' }; let key = 'name'; console.log(obj[key]); // outputs 'JavaScript'
Nested objects and variable keys
For nested objects, you can chain square brackets to access deeper properties using variable keys.
let obj = { user: { id: 1, profile: { username: 'jsmith' } } }; let outerKey = 'user'; let innerKey = 'profile'; let finalKey = 'username'; console.log(obj[outerKey][innerKey][finalKey]); // outputs 'jsmith'
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

Using Object.defineProperty
with variable keys
Object.defineProperty
can be used for more control over property behavior, and it also accepts variable keys.
let obj = {}; let key = 'id'; Object.defineProperty(obj, key, { value: 1, writable: true, enumerable: true, configurable: true }); console.log(obj.id); // outputs 1
ES6 Map
object with variable keys
If you need a key that isn't a string or symbol, consider using a Map
, which can take any value as a key.
let map = new Map(); let keyObj = {}; map.set(keyObj, 'value'); console.log(map.get(keyObj)); // outputs 'value'
Dealing with variable keys in object destructuring
Variable keys can be used with the destructuring syntax in ES6 to extract properties from objects into variables with dynamic names.
const keyVar = 'name'; const { [keyVar]: nameValue } = { name: 'JavaScript' }; console.log(nameValue); // 'JavaScript'
Addressing non-existent keys
Attempting to access a property with a key stored in a variable that does not exist on the object will return undefined
. Use the in
operator or hasOwnProperty
method to check for the existence of a key.
let obj = { name: 'Alice' }; let key = 'age'; console.log(key in obj); // false console.log(obj.hasOwnProperty(key)); // false
Dynamic property access with Proxies
The Proxy
object is used to define custom behavior for fundamental operations, such as property lookup, assignment, and enumeration, which can be conditional based on variable keys.
let key = 'dynamicKey'; let proxy = new Proxy({}, { get(target, propKey, receiver) { if (propKey === key) { return 'intercepted value'; } return Reflect.get(target, propKey, receiver); } }); console.log(proxy.dynamicKey); // 'intercepted value'
Considerations for serialization and performance
When serializing objects with variable keys, remember that JSON.stringify will only include string keys. Symbol or function keys will be omitted from the serialized output.
When dealing with objects that frequently change keys or have a vast number of keys, it's essential to be mindful of the performance implications. Objects are not optimized for high-frequency dynamic key operations, which can lead to deoptimization and increased memory usage.
Best practices for using variable keys
- Variable names used as keys should be self-descriptive to ensure maintainability.
- Consider using
Map
for scenarios where keys are objects or when key values change dynamically. - Guard against potential key collisions by checking for the existence of keys before assigning them.
- Be aware of the implications when using variable keys with serialization and performance.
TOC
November 7, 2023
Using a variable as a key in JavaScript allows for dynamic assignment and access to properties within objects. It's a flexible way to handle property identifiers that are determined at runtime.
Understanding objects and keys in JavaScript
Objects in JavaScript are collections of properties, and properties are key-value pairs. A key (or property name) can be any string or symbol, while the value can be any valid JavaScript value.
let obj = { key: 'value' };
Defining a variable as a key
You can define a variable to use as a key using square brackets []
in an object literal. This is known as computed property names.
let keyName = 'dynamicKey'; let obj = { [keyName]: 'value' }; console.log(obj.dynamicKey); // outputs 'value'
Using variables for dynamic properties
When creating an object, if the property names are not known until runtime, you can use a variable as the key.
function createObject(key, value) { return { [key]: value }; } let obj = createObject('id', 1); console.log(obj.id); // outputs 1
Modifying object properties with variable keys
To modify the value of a property using a variable key, use the variable within square brackets to access the property.
let obj = { id: 1 }; let keyName = 'id'; obj[keyName] = 2; console.log(obj.id); // outputs 2
Accessing properties dynamically
You can also use a variable to access properties of an object dynamically.
let obj = { id: 1, name: 'JavaScript' }; let key = 'name'; console.log(obj[key]); // outputs 'JavaScript'
Nested objects and variable keys
For nested objects, you can chain square brackets to access deeper properties using variable keys.
let obj = { user: { id: 1, profile: { username: 'jsmith' } } }; let outerKey = 'user'; let innerKey = 'profile'; let finalKey = 'username'; console.log(obj[outerKey][innerKey][finalKey]); // outputs 'jsmith'
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

Using Object.defineProperty
with variable keys
Object.defineProperty
can be used for more control over property behavior, and it also accepts variable keys.
let obj = {}; let key = 'id'; Object.defineProperty(obj, key, { value: 1, writable: true, enumerable: true, configurable: true }); console.log(obj.id); // outputs 1
ES6 Map
object with variable keys
If you need a key that isn't a string or symbol, consider using a Map
, which can take any value as a key.
let map = new Map(); let keyObj = {}; map.set(keyObj, 'value'); console.log(map.get(keyObj)); // outputs 'value'
Dealing with variable keys in object destructuring
Variable keys can be used with the destructuring syntax in ES6 to extract properties from objects into variables with dynamic names.
const keyVar = 'name'; const { [keyVar]: nameValue } = { name: 'JavaScript' }; console.log(nameValue); // 'JavaScript'
Addressing non-existent keys
Attempting to access a property with a key stored in a variable that does not exist on the object will return undefined
. Use the in
operator or hasOwnProperty
method to check for the existence of a key.
let obj = { name: 'Alice' }; let key = 'age'; console.log(key in obj); // false console.log(obj.hasOwnProperty(key)); // false
Dynamic property access with Proxies
The Proxy
object is used to define custom behavior for fundamental operations, such as property lookup, assignment, and enumeration, which can be conditional based on variable keys.
let key = 'dynamicKey'; let proxy = new Proxy({}, { get(target, propKey, receiver) { if (propKey === key) { return 'intercepted value'; } return Reflect.get(target, propKey, receiver); } }); console.log(proxy.dynamicKey); // 'intercepted value'
Considerations for serialization and performance
When serializing objects with variable keys, remember that JSON.stringify will only include string keys. Symbol or function keys will be omitted from the serialized output.
When dealing with objects that frequently change keys or have a vast number of keys, it's essential to be mindful of the performance implications. Objects are not optimized for high-frequency dynamic key operations, which can lead to deoptimization and increased memory usage.
Best practices for using variable keys
- Variable names used as keys should be self-descriptive to ensure maintainability.
- Consider using
Map
for scenarios where keys are objects or when key values change dynamically. - Guard against potential key collisions by checking for the existence of keys before assigning them.
- Be aware of the implications when using variable keys with serialization and performance.
November 7, 2023
Using a variable as a key in JavaScript allows for dynamic assignment and access to properties within objects. It's a flexible way to handle property identifiers that are determined at runtime.
Understanding objects and keys in JavaScript
Objects in JavaScript are collections of properties, and properties are key-value pairs. A key (or property name) can be any string or symbol, while the value can be any valid JavaScript value.
let obj = { key: 'value' };
Defining a variable as a key
You can define a variable to use as a key using square brackets []
in an object literal. This is known as computed property names.
let keyName = 'dynamicKey'; let obj = { [keyName]: 'value' }; console.log(obj.dynamicKey); // outputs 'value'
Using variables for dynamic properties
When creating an object, if the property names are not known until runtime, you can use a variable as the key.
function createObject(key, value) { return { [key]: value }; } let obj = createObject('id', 1); console.log(obj.id); // outputs 1
Modifying object properties with variable keys
To modify the value of a property using a variable key, use the variable within square brackets to access the property.
let obj = { id: 1 }; let keyName = 'id'; obj[keyName] = 2; console.log(obj.id); // outputs 2
Accessing properties dynamically
You can also use a variable to access properties of an object dynamically.
let obj = { id: 1, name: 'JavaScript' }; let key = 'name'; console.log(obj[key]); // outputs 'JavaScript'
Nested objects and variable keys
For nested objects, you can chain square brackets to access deeper properties using variable keys.
let obj = { user: { id: 1, profile: { username: 'jsmith' } } }; let outerKey = 'user'; let innerKey = 'profile'; let finalKey = 'username'; console.log(obj[outerKey][innerKey][finalKey]); // outputs 'jsmith'
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

Using Object.defineProperty
with variable keys
Object.defineProperty
can be used for more control over property behavior, and it also accepts variable keys.
let obj = {}; let key = 'id'; Object.defineProperty(obj, key, { value: 1, writable: true, enumerable: true, configurable: true }); console.log(obj.id); // outputs 1
ES6 Map
object with variable keys
If you need a key that isn't a string or symbol, consider using a Map
, which can take any value as a key.
let map = new Map(); let keyObj = {}; map.set(keyObj, 'value'); console.log(map.get(keyObj)); // outputs 'value'
Dealing with variable keys in object destructuring
Variable keys can be used with the destructuring syntax in ES6 to extract properties from objects into variables with dynamic names.
const keyVar = 'name'; const { [keyVar]: nameValue } = { name: 'JavaScript' }; console.log(nameValue); // 'JavaScript'
Addressing non-existent keys
Attempting to access a property with a key stored in a variable that does not exist on the object will return undefined
. Use the in
operator or hasOwnProperty
method to check for the existence of a key.
let obj = { name: 'Alice' }; let key = 'age'; console.log(key in obj); // false console.log(obj.hasOwnProperty(key)); // false
Dynamic property access with Proxies
The Proxy
object is used to define custom behavior for fundamental operations, such as property lookup, assignment, and enumeration, which can be conditional based on variable keys.
let key = 'dynamicKey'; let proxy = new Proxy({}, { get(target, propKey, receiver) { if (propKey === key) { return 'intercepted value'; } return Reflect.get(target, propKey, receiver); } }); console.log(proxy.dynamicKey); // 'intercepted value'
Considerations for serialization and performance
When serializing objects with variable keys, remember that JSON.stringify will only include string keys. Symbol or function keys will be omitted from the serialized output.
When dealing with objects that frequently change keys or have a vast number of keys, it's essential to be mindful of the performance implications. Objects are not optimized for high-frequency dynamic key operations, which can lead to deoptimization and increased memory usage.
Best practices for using variable keys
- Variable names used as keys should be self-descriptive to ensure maintainability.
- Consider using
Map
for scenarios where keys are objects or when key values change dynamically. - Guard against potential key collisions by checking for the existence of keys before assigning them.
- Be aware of the implications when using variable keys with serialization and performance.
What is Basedash?
What is Basedash?
What is Basedash?
Ship faster, worry less with Basedash
Ship faster, worry less with Basedash
Ship faster, worry less with Basedash
You're busy enough with product work to be weighed down building, maintaining, scoping and developing internal apps and admin panels. Forget all of that, and give your team the admin panel that you don't have to build. Launch in less time than it takes to run a standup.
You're busy enough with product work to be weighed down building, maintaining, scoping and developing internal apps and admin panels. Forget all of that, and give your team the admin panel that you don't have to build. Launch in less time than it takes to run a standup.
You're busy enough with product work to be weighed down building, maintaining, scoping and developing internal apps and admin panels. Forget all of that, and give your team the admin panel that you don't have to build. Launch in less time than it takes to run a standup.




Dashboards and charts
Edit data, create records, oversee how your product is running without the need to build or manage custom software.
USER CRM
ADMIN PANEL
SQL COMPOSER WITH AI

Related posts
Related posts
Related posts



How to Remove Characters from a String in JavaScript
Jeremy Sarchet



How to Sort Strings in JavaScript
Max Musing



How to Remove Spaces from a String in JavaScript
Jeremy Sarchet



Detecting Prime Numbers in JavaScript
Robert Cooper



How to Parse Boolean Values in JavaScript
Max Musing



How to Remove a Substring from a String in JavaScript
Robert Cooper