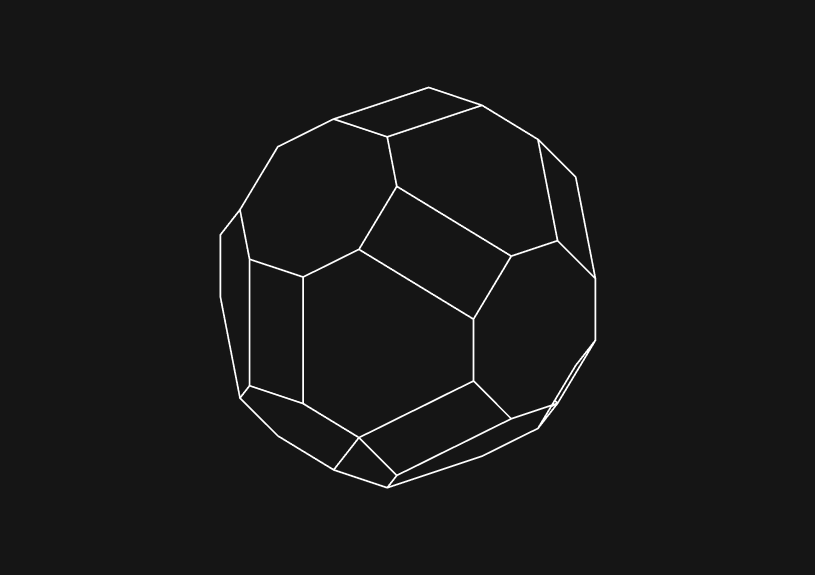
How to check if value is in enum in TypeScript
October 27, 2023
In TypeScript, enums allow you to define named sets of numeric or string values. Sometimes, you'll want to determine if a specific value exists within an enum. Here's how to do that efficiently.
Enum basics
Before diving into checking values, it's important to understand the structure of enums. In TypeScript, an enum can be numeric or string-based.
enum Color { Red, Green, Blue } enum NamedColor { Red = "RED", Green = "GREEN", Blue = "BLUE" }
Checking if a numeric value is in an enum
To verify if a numeric value is part of an enum, you can use the in
keyword, combined with the enum's name.
const valueToCheck = 1; if (valueToCheck in Color) { console.log("Value is in enum"); } else { console.log("Value is not in enum"); }
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

Checking if a string value is in an enum
For string-based enums, you can still use the in
keyword. But, to ensure that you're only checking against the enum's actual values (and not its reverse mapping), you'll need to utilize the Object.values
method.
const stringToCheck = "RED"; if (Object.values(NamedColor).includes(stringToCheck)) { console.log("String is in enum"); } else { console.log("String is not in enum"); }
Guarding against reverse mapping
TypeScript provides reverse mapping for numeric enums. This means you can access the name of an enum member given its value. However, this also means you need to be careful when checking if a string is in a numeric enum.
console.log(Color[Color.Red]); // Outputs: "Red" const stringToCheck = "Red"; if (stringToCheck in Color) { console.log("String is in enum"); // This will be true, due to reverse mapping } else { console.log("String is not in enum"); }
To guard against this, ensure that the value you're checking is not a number.
if (isNaN(Number(stringToCheck)) && stringToCheck in Color) { console.log("String is in enum"); } else { console.log("String is not in enum"); }
Using helper functions
For repeated checks, you might consider wrapping the above logic in helper functions.
function isInNumericEnum(value: number, enumeration: any): boolean { return value in enumeration; } function isInStringEnum(value: string, enumeration: any): boolean { return Object.values(enumeration).includes(value); } // Usage console.log(isInNumericEnum(1, Color)); // true console.log(isInStringEnum("RED", NamedColor)); // true
By following the above approaches, you can confidently check if values exist within your TypeScript enums, ensuring cleaner and more maintainable code.
TOC
October 27, 2023
In TypeScript, enums allow you to define named sets of numeric or string values. Sometimes, you'll want to determine if a specific value exists within an enum. Here's how to do that efficiently.
Enum basics
Before diving into checking values, it's important to understand the structure of enums. In TypeScript, an enum can be numeric or string-based.
enum Color { Red, Green, Blue } enum NamedColor { Red = "RED", Green = "GREEN", Blue = "BLUE" }
Checking if a numeric value is in an enum
To verify if a numeric value is part of an enum, you can use the in
keyword, combined with the enum's name.
const valueToCheck = 1; if (valueToCheck in Color) { console.log("Value is in enum"); } else { console.log("Value is not in enum"); }
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

Checking if a string value is in an enum
For string-based enums, you can still use the in
keyword. But, to ensure that you're only checking against the enum's actual values (and not its reverse mapping), you'll need to utilize the Object.values
method.
const stringToCheck = "RED"; if (Object.values(NamedColor).includes(stringToCheck)) { console.log("String is in enum"); } else { console.log("String is not in enum"); }
Guarding against reverse mapping
TypeScript provides reverse mapping for numeric enums. This means you can access the name of an enum member given its value. However, this also means you need to be careful when checking if a string is in a numeric enum.
console.log(Color[Color.Red]); // Outputs: "Red" const stringToCheck = "Red"; if (stringToCheck in Color) { console.log("String is in enum"); // This will be true, due to reverse mapping } else { console.log("String is not in enum"); }
To guard against this, ensure that the value you're checking is not a number.
if (isNaN(Number(stringToCheck)) && stringToCheck in Color) { console.log("String is in enum"); } else { console.log("String is not in enum"); }
Using helper functions
For repeated checks, you might consider wrapping the above logic in helper functions.
function isInNumericEnum(value: number, enumeration: any): boolean { return value in enumeration; } function isInStringEnum(value: string, enumeration: any): boolean { return Object.values(enumeration).includes(value); } // Usage console.log(isInNumericEnum(1, Color)); // true console.log(isInStringEnum("RED", NamedColor)); // true
By following the above approaches, you can confidently check if values exist within your TypeScript enums, ensuring cleaner and more maintainable code.
October 27, 2023
In TypeScript, enums allow you to define named sets of numeric or string values. Sometimes, you'll want to determine if a specific value exists within an enum. Here's how to do that efficiently.
Enum basics
Before diving into checking values, it's important to understand the structure of enums. In TypeScript, an enum can be numeric or string-based.
enum Color { Red, Green, Blue } enum NamedColor { Red = "RED", Green = "GREEN", Blue = "BLUE" }
Checking if a numeric value is in an enum
To verify if a numeric value is part of an enum, you can use the in
keyword, combined with the enum's name.
const valueToCheck = 1; if (valueToCheck in Color) { console.log("Value is in enum"); } else { console.log("Value is not in enum"); }
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

Checking if a string value is in an enum
For string-based enums, you can still use the in
keyword. But, to ensure that you're only checking against the enum's actual values (and not its reverse mapping), you'll need to utilize the Object.values
method.
const stringToCheck = "RED"; if (Object.values(NamedColor).includes(stringToCheck)) { console.log("String is in enum"); } else { console.log("String is not in enum"); }
Guarding against reverse mapping
TypeScript provides reverse mapping for numeric enums. This means you can access the name of an enum member given its value. However, this also means you need to be careful when checking if a string is in a numeric enum.
console.log(Color[Color.Red]); // Outputs: "Red" const stringToCheck = "Red"; if (stringToCheck in Color) { console.log("String is in enum"); // This will be true, due to reverse mapping } else { console.log("String is not in enum"); }
To guard against this, ensure that the value you're checking is not a number.
if (isNaN(Number(stringToCheck)) && stringToCheck in Color) { console.log("String is in enum"); } else { console.log("String is not in enum"); }
Using helper functions
For repeated checks, you might consider wrapping the above logic in helper functions.
function isInNumericEnum(value: number, enumeration: any): boolean { return value in enumeration; } function isInStringEnum(value: string, enumeration: any): boolean { return Object.values(enumeration).includes(value); } // Usage console.log(isInNumericEnum(1, Color)); // true console.log(isInStringEnum("RED", NamedColor)); // true
By following the above approaches, you can confidently check if values exist within your TypeScript enums, ensuring cleaner and more maintainable code.
What is Basedash?
What is Basedash?
What is Basedash?
Ship faster, worry less with Basedash
Ship faster, worry less with Basedash
You're busy enough with product work to be weighed down building, maintaining, scoping and developing internal apps and admin panels. Forget all of that, and give your team the admin panel that you don't have to build. Launch in less time than it takes to run a standup.
You're busy enough with product work to be weighed down building, maintaining, scoping and developing internal apps and admin panels. Forget all of that, and give your team the admin panel that you don't have to build. Launch in less time than it takes to run a standup.
You're busy enough with product work to be weighed down building, maintaining, scoping and developing internal apps and admin panels. Forget all of that, and give your team the admin panel that you don't have to build. Launch in less time than it takes to run a standup.




Dashboards and charts
Edit data, create records, oversee how your product is running without the need to build or manage custom software.
USER CRM
ADMIN PANEL
SQL COMPOSER WITH AI

Related posts
Related posts
Related posts



How to turn webpages into editable canvases with a JavaScript bookmarklet
Kris Lachance



How to fix the "not all code paths return a value" issue in TypeScript
Kris Lachance



Working with WebSockets in Node.js using TypeScript
Kris Lachance



Type Annotations Can Only Be Used in TypeScript Files
Kris Lachance



Guide to TypeScript Recursive Type
Kris Lachance



How to Configure Knex.js with TypeScript
Kris Lachance