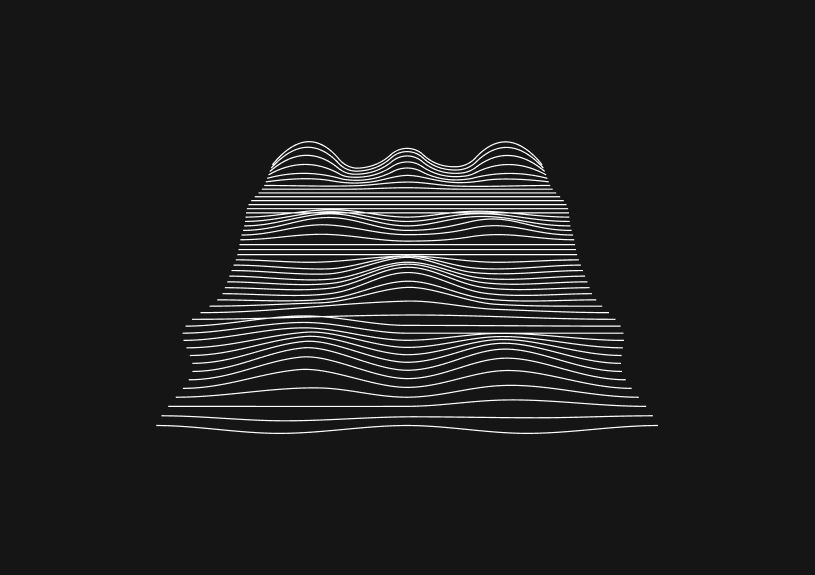
How to Compare Strings in TypeScript
October 27, 2023
In TypeScript, as in JavaScript, strings can be compared for equality and ordering. This guide will take you through various methods and best practices for comparing strings.
Basic Equality Comparison
The simplest way to compare strings is using the ===
(strict equality) operator. This checks if the strings are of the same value and type.
const str1: string = "hello"; const str2: string = "hello"; const str3: string = "world"; console.log(str1 === str2); // true console.log(str1 === str3); // false
Case-Insensitive Comparison
Sometimes, we want to compare strings in a case-insensitive manner. A common approach is to convert both strings to the same case (either upper or lower) before comparison.
const str1: string = "HELLO"; const str2: string = "hello"; console.log(str1.toLowerCase() === str2.toLowerCase()); // true
Locale-aware Comparison
For strings that may contain characters from various languages, a locale-aware comparison is essential. Use the localeCompare()
method:
const germanStr1: string = "ä"; const germanStr2: string = "z"; console.log(germanStr1.localeCompare(germanStr2)); // -1 because "ä" comes before "z" in German sorting
The localeCompare()
method returns:
0
if the strings are equal- A negative number if the reference string comes before the compare string
- A positive number if the reference string comes after the compare string
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

Comparing String Lengths
To compare the lengths of strings, you can simply use the length property of the string.
const str1: string = "apple"; const str2: string = "bananas"; console.log(str1.length === str2.length); // false console.log(str1.length > str2.length); // false console.log(str1.length < str2.length); // true
Using the startsWith()
and endsWith()
Methods
These methods allow you to check if a string starts or ends with a specific substring.
const mainStr: string = "TypeScript is great!"; console.log(mainStr.startsWith("Type")); // true console.log(mainStr.endsWith("!")); // true
Handling null
and undefined
If there's a possibility that your string variables might be null
or undefined
, it's a good practice to handle these scenarios before comparison.
const str1: string | null | undefined = getSomeString(); const str2: string = "test"; if(str1 && str1 === str2) { console.log("Strings are equal"); } else { console.log("Strings are not equal or str1 is null/undefined"); }
In this guide, we explored various methods to compare strings in TypeScript. Depending on the specific needs of your application, you can pick the method that suits best. From basic equality checks to more nuanced locale-aware comparisons, TypeScript offers flexible tools for string comparisons.
TOC
October 27, 2023
In TypeScript, as in JavaScript, strings can be compared for equality and ordering. This guide will take you through various methods and best practices for comparing strings.
Basic Equality Comparison
The simplest way to compare strings is using the ===
(strict equality) operator. This checks if the strings are of the same value and type.
const str1: string = "hello"; const str2: string = "hello"; const str3: string = "world"; console.log(str1 === str2); // true console.log(str1 === str3); // false
Case-Insensitive Comparison
Sometimes, we want to compare strings in a case-insensitive manner. A common approach is to convert both strings to the same case (either upper or lower) before comparison.
const str1: string = "HELLO"; const str2: string = "hello"; console.log(str1.toLowerCase() === str2.toLowerCase()); // true
Locale-aware Comparison
For strings that may contain characters from various languages, a locale-aware comparison is essential. Use the localeCompare()
method:
const germanStr1: string = "ä"; const germanStr2: string = "z"; console.log(germanStr1.localeCompare(germanStr2)); // -1 because "ä" comes before "z" in German sorting
The localeCompare()
method returns:
0
if the strings are equal- A negative number if the reference string comes before the compare string
- A positive number if the reference string comes after the compare string
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

Comparing String Lengths
To compare the lengths of strings, you can simply use the length property of the string.
const str1: string = "apple"; const str2: string = "bananas"; console.log(str1.length === str2.length); // false console.log(str1.length > str2.length); // false console.log(str1.length < str2.length); // true
Using the startsWith()
and endsWith()
Methods
These methods allow you to check if a string starts or ends with a specific substring.
const mainStr: string = "TypeScript is great!"; console.log(mainStr.startsWith("Type")); // true console.log(mainStr.endsWith("!")); // true
Handling null
and undefined
If there's a possibility that your string variables might be null
or undefined
, it's a good practice to handle these scenarios before comparison.
const str1: string | null | undefined = getSomeString(); const str2: string = "test"; if(str1 && str1 === str2) { console.log("Strings are equal"); } else { console.log("Strings are not equal or str1 is null/undefined"); }
In this guide, we explored various methods to compare strings in TypeScript. Depending on the specific needs of your application, you can pick the method that suits best. From basic equality checks to more nuanced locale-aware comparisons, TypeScript offers flexible tools for string comparisons.
October 27, 2023
In TypeScript, as in JavaScript, strings can be compared for equality and ordering. This guide will take you through various methods and best practices for comparing strings.
Basic Equality Comparison
The simplest way to compare strings is using the ===
(strict equality) operator. This checks if the strings are of the same value and type.
const str1: string = "hello"; const str2: string = "hello"; const str3: string = "world"; console.log(str1 === str2); // true console.log(str1 === str3); // false
Case-Insensitive Comparison
Sometimes, we want to compare strings in a case-insensitive manner. A common approach is to convert both strings to the same case (either upper or lower) before comparison.
const str1: string = "HELLO"; const str2: string = "hello"; console.log(str1.toLowerCase() === str2.toLowerCase()); // true
Locale-aware Comparison
For strings that may contain characters from various languages, a locale-aware comparison is essential. Use the localeCompare()
method:
const germanStr1: string = "ä"; const germanStr2: string = "z"; console.log(germanStr1.localeCompare(germanStr2)); // -1 because "ä" comes before "z" in German sorting
The localeCompare()
method returns:
0
if the strings are equal- A negative number if the reference string comes before the compare string
- A positive number if the reference string comes after the compare string
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

Comparing String Lengths
To compare the lengths of strings, you can simply use the length property of the string.
const str1: string = "apple"; const str2: string = "bananas"; console.log(str1.length === str2.length); // false console.log(str1.length > str2.length); // false console.log(str1.length < str2.length); // true
Using the startsWith()
and endsWith()
Methods
These methods allow you to check if a string starts or ends with a specific substring.
const mainStr: string = "TypeScript is great!"; console.log(mainStr.startsWith("Type")); // true console.log(mainStr.endsWith("!")); // true
Handling null
and undefined
If there's a possibility that your string variables might be null
or undefined
, it's a good practice to handle these scenarios before comparison.
const str1: string | null | undefined = getSomeString(); const str2: string = "test"; if(str1 && str1 === str2) { console.log("Strings are equal"); } else { console.log("Strings are not equal or str1 is null/undefined"); }
In this guide, we explored various methods to compare strings in TypeScript. Depending on the specific needs of your application, you can pick the method that suits best. From basic equality checks to more nuanced locale-aware comparisons, TypeScript offers flexible tools for string comparisons.
What is Basedash?
What is Basedash?
What is Basedash?
Ship faster, worry less with Basedash
Ship faster, worry less with Basedash
You're busy enough with product work to be weighed down building, maintaining, scoping and developing internal apps and admin panels. Forget all of that, and give your team the admin panel that you don't have to build. Launch in less time than it takes to run a standup.
You're busy enough with product work to be weighed down building, maintaining, scoping and developing internal apps and admin panels. Forget all of that, and give your team the admin panel that you don't have to build. Launch in less time than it takes to run a standup.
You're busy enough with product work to be weighed down building, maintaining, scoping and developing internal apps and admin panels. Forget all of that, and give your team the admin panel that you don't have to build. Launch in less time than it takes to run a standup.




Dashboards and charts
Edit data, create records, oversee how your product is running without the need to build or manage custom software.
USER CRM
ADMIN PANEL
SQL COMPOSER WITH AI

Related posts
Related posts
Related posts



How to turn webpages into editable canvases with a JavaScript bookmarklet
Kris Lachance



How to fix the "not all code paths return a value" issue in TypeScript
Kris Lachance



Working with WebSockets in Node.js using TypeScript
Kris Lachance



Type Annotations Can Only Be Used in TypeScript Files
Kris Lachance



Guide to TypeScript Recursive Type
Kris Lachance



How to Configure Knex.js with TypeScript
Kris Lachance