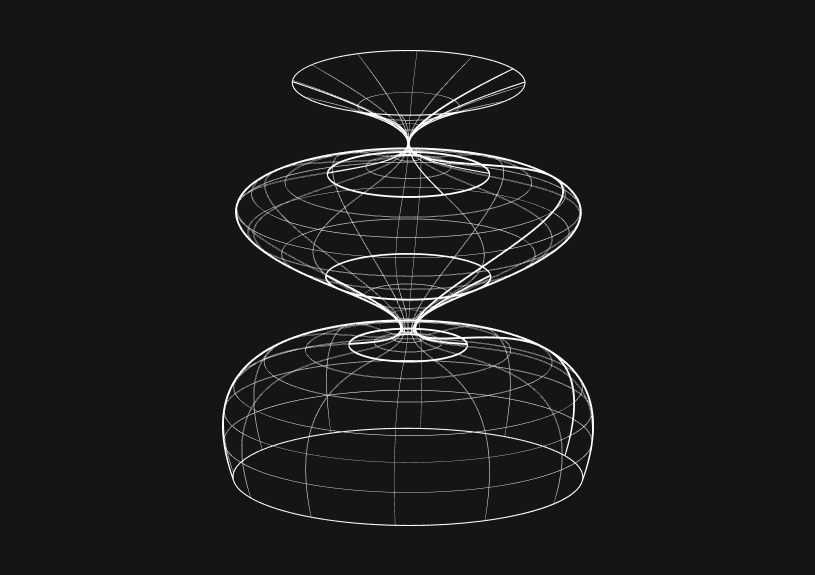
How to print a new line in TypeScript
October 27, 2023
In TypeScript, printing a new line to the console or incorporating a new line into a string is straightforward. This guide will show you different methods to achieve this.
Using \n
for a new line
The most common method to insert a new line in a string in TypeScript (and in most programming languages) is by using the newline character \n
.
In a console log:
console.log("This is the first line.\nThis is the second line.");
When executed, this will display:
This is the first line. This is the second line.
In a string variable:
let multiLineString = "Hello,\nWorld!"; console.log(multiLineString);
Using template literals
Introduced in ECMAScript 2015 (ES6), template literals provide a convenient way to create multi-line strings without needing to use the \\n
character. They're wrapped in backticks (``) instead of the usual single (' ') or double (" ") quotes.
let multiLineString = `This is the first line. This is the second line.`; console.log(multiLineString);
Printing to HTML content
If you're using TypeScript with a web framework and you want to insert a new line into HTML content, the newline character \n
won't work. Instead, you'd use the HTML line break tag <br>
.
let htmlString = "This is the first line.<br>This is the second line."; document.getElementById("myElement").innerHTML = htmlString;
String concatenation
You can also concatenate strings and the newline character \n
to produce multi-line strings:
let line1 = "This is the first line."; let line2 = "This is the second line."; let multiLineString = line1 + "\n" + line2; console.log(multiLineString);
Final thoughts
Printing a new line in TypeScript is as easy as knowing which character or method to use based on your application's needs. Whether you're printing to the console, creating a string variable, or interacting with the DOM, you have several methods at your disposal to effectively manage new lines.
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

TOC
October 27, 2023
In TypeScript, printing a new line to the console or incorporating a new line into a string is straightforward. This guide will show you different methods to achieve this.
Using \n
for a new line
The most common method to insert a new line in a string in TypeScript (and in most programming languages) is by using the newline character \n
.
In a console log:
console.log("This is the first line.\nThis is the second line.");
When executed, this will display:
This is the first line. This is the second line.
In a string variable:
let multiLineString = "Hello,\nWorld!"; console.log(multiLineString);
Using template literals
Introduced in ECMAScript 2015 (ES6), template literals provide a convenient way to create multi-line strings without needing to use the \\n
character. They're wrapped in backticks (``) instead of the usual single (' ') or double (" ") quotes.
let multiLineString = `This is the first line. This is the second line.`; console.log(multiLineString);
Printing to HTML content
If you're using TypeScript with a web framework and you want to insert a new line into HTML content, the newline character \n
won't work. Instead, you'd use the HTML line break tag <br>
.
let htmlString = "This is the first line.<br>This is the second line."; document.getElementById("myElement").innerHTML = htmlString;
String concatenation
You can also concatenate strings and the newline character \n
to produce multi-line strings:
let line1 = "This is the first line."; let line2 = "This is the second line."; let multiLineString = line1 + "\n" + line2; console.log(multiLineString);
Final thoughts
Printing a new line in TypeScript is as easy as knowing which character or method to use based on your application's needs. Whether you're printing to the console, creating a string variable, or interacting with the DOM, you have several methods at your disposal to effectively manage new lines.
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

October 27, 2023
In TypeScript, printing a new line to the console or incorporating a new line into a string is straightforward. This guide will show you different methods to achieve this.
Using \n
for a new line
The most common method to insert a new line in a string in TypeScript (and in most programming languages) is by using the newline character \n
.
In a console log:
console.log("This is the first line.\nThis is the second line.");
When executed, this will display:
This is the first line. This is the second line.
In a string variable:
let multiLineString = "Hello,\nWorld!"; console.log(multiLineString);
Using template literals
Introduced in ECMAScript 2015 (ES6), template literals provide a convenient way to create multi-line strings without needing to use the \\n
character. They're wrapped in backticks (``) instead of the usual single (' ') or double (" ") quotes.
let multiLineString = `This is the first line. This is the second line.`; console.log(multiLineString);
Printing to HTML content
If you're using TypeScript with a web framework and you want to insert a new line into HTML content, the newline character \n
won't work. Instead, you'd use the HTML line break tag <br>
.
let htmlString = "This is the first line.<br>This is the second line."; document.getElementById("myElement").innerHTML = htmlString;
String concatenation
You can also concatenate strings and the newline character \n
to produce multi-line strings:
let line1 = "This is the first line."; let line2 = "This is the second line."; let multiLineString = line1 + "\n" + line2; console.log(multiLineString);
Final thoughts
Printing a new line in TypeScript is as easy as knowing which character or method to use based on your application's needs. Whether you're printing to the console, creating a string variable, or interacting with the DOM, you have several methods at your disposal to effectively manage new lines.
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

What is Basedash?
What is Basedash?
What is Basedash?
Ship faster, worry less with Basedash
Ship faster, worry less with Basedash
You're busy enough with product work to be weighed down building, maintaining, scoping and developing internal apps and admin panels. Forget all of that, and give your team the admin panel that you don't have to build. Launch in less time than it takes to run a standup.
You're busy enough with product work to be weighed down building, maintaining, scoping and developing internal apps and admin panels. Forget all of that, and give your team the admin panel that you don't have to build. Launch in less time than it takes to run a standup.
You're busy enough with product work to be weighed down building, maintaining, scoping and developing internal apps and admin panels. Forget all of that, and give your team the admin panel that you don't have to build. Launch in less time than it takes to run a standup.




Dashboards and charts
Edit data, create records, oversee how your product is running without the need to build or manage custom software.
USER CRM
ADMIN PANEL
SQL COMPOSER WITH AI

Related posts
Related posts
Related posts



How to turn webpages into editable canvases with a JavaScript bookmarklet
Kris Lachance



How to fix the "not all code paths return a value" issue in TypeScript
Kris Lachance



Working with WebSockets in Node.js using TypeScript
Kris Lachance



Type Annotations Can Only Be Used in TypeScript Files
Kris Lachance



Guide to TypeScript Recursive Type
Kris Lachance



How to Configure Knex.js with TypeScript
Kris Lachance