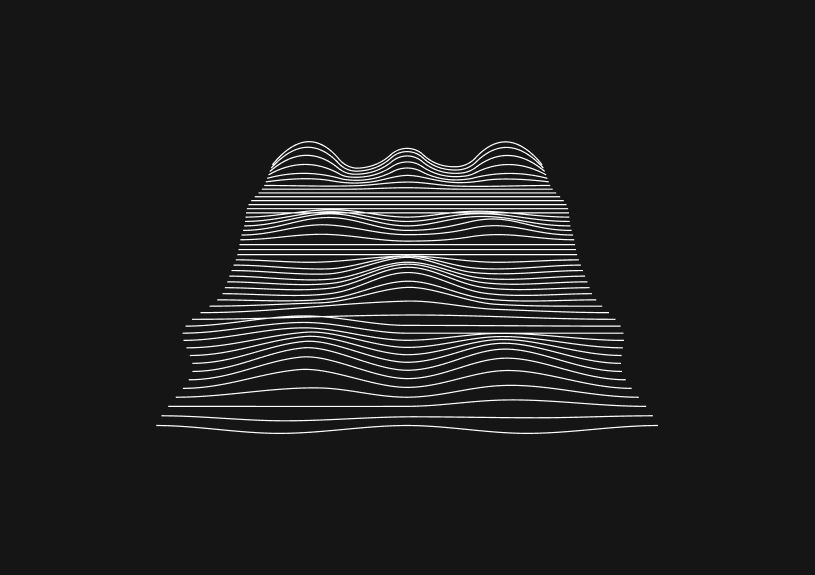
Pagination in React Query: A Guide
January 29, 2024
Pagination is a common requirement in modern web applications, and React Query offers a straightforward way to implement it. In this guide we’ll walk you through setting up pagination using React Query.
What is pagination in React Query?
Pagination involves splitting a large dataset into smaller, manageable chunks, typically displayed as pages. React Query handles pagination by providing built-in hooks that manage the data fetching and state management aspects. It offers flexibility to implement different types of pagination, like traditional numbered pages or infinite scrolling.
Set up the environment
First you should make sure you have React Query installed in your project:
npm install react-query
Then you’ll need to import the necessary hooks and client from React Query:
import { useQuery, QueryClient, QueryClientProvider } from 'react-query';
How to set up pagination in React Query
To begin with pagination, you need a function to fetch your data. This function typically takes a page number as an argument:
const fetchProjects = async (page = 0) => { const res = await fetch('/api/projects?page=' + page); return res.json(); };
Use the useQuery
hook to fetch data for a specific page:
const Projects = ({ page }) => { const { data, isLoading, isError } = useQuery(['projects', page], () => fetchProjects(page)); // Render your component based on the data };
How to handle page changes
To navigate between pages, you need to manage the page
state and pass it to your query:
const PaginatedProjects = () => { const [page, setPage] = useState(0); return ( <div> <Projects page={page} /> <button onClick={() => setPage(old => Math.max(old - 1, 0))}>Previous Page</button> <button onClick={() => setPage(old => old + 1)}>Next Page</button> </div> ); };
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

How to handle infinite scrolling in react query
If you prefer infinite scrolling over traditional pagination, use useInfiniteQuery
:
const fetchProjectsInfinite = ({ pageParam = 0 }) => { return fetchProjects(pageParam); }; const InfiniteProjects = () => { const { data, fetchNextPage, hasNextPage, isFetchingNextPage, } = useInfiniteQuery('projects', fetchProjectsInfinite, { getNextPageParam: (lastPage, pages) => lastPage.nextPage, }); // Render your component with infinite scroll functionality };
Optimize with React Query’s cache
React Query caches your queries, which means when a user navigates back to a previously visited page, the data is served from the cache, reducing loading times and server requests.
Integrate with external tools
If your project requires an admin panel to view and edit data from your database, consider using tools like Basedash. It allows you to generate admin panels and manage database data efficiently, which can be especially handy when dealing with large datasets in paginated queries.
How to use keepPreviousData
The keepPreviousData
option in React Query is a game-changer for improving user experience in pagination. It allows your application to retain data from a previous page while new data is being loaded. This feature is essential for smoother page transitions and reducing the perceived loading time.
Why you should use keepPreviousData
- Seamless Transitions: By keeping the data of the previous page visible until the new page's data loads, users experience less disruption. This eliminates the jarring effect of a blank screen or a loading spinner during page switches.
- Maintain Scroll Position: Particularly useful in infinite scrolling scenarios, where you want to keep the user's scroll position stable as new data loads.
- Optimized User Experience: It provides a more continuous and fluid browsing experience, which is crucial for keeping users engaged, especially in data-heavy applications.
How to implement keepPreviousData
It’s pretty straightforward to implement. Here's how you can modify the useQuery
hook for paginated data:
const { data, isLoading, isError } = useQuery( ['projects', page], () => fetchProjects(page), { keepPreviousData: true } );
In this example, when the page
state changes (as the user navigates to a different page), the old data (from the previous page) is rendered until the new data is fetched. This usually leads to a better user experience since you’re reducing the abrupt changes that you’ll usually see during data fetching.
Practical Example
Consider a list of projects that users can browse through multiple pages. When a user clicks "Next Page," instead of immediately showing a loading indicator or a blank page, the application continues to show the current page's content. Behind the scenes, the new page's data is being fetched. Once the data is available, the UI updates seamlessly, reducing the cognitive load and disorientation associated with traditional pagination methods.
How to handle loading and error states
Always handle loading and error states in your components:
if (isLoading) return 'Loading...'; if (isError) return 'An error has occurred';
TOC
January 29, 2024
Pagination is a common requirement in modern web applications, and React Query offers a straightforward way to implement it. In this guide we’ll walk you through setting up pagination using React Query.
What is pagination in React Query?
Pagination involves splitting a large dataset into smaller, manageable chunks, typically displayed as pages. React Query handles pagination by providing built-in hooks that manage the data fetching and state management aspects. It offers flexibility to implement different types of pagination, like traditional numbered pages or infinite scrolling.
Set up the environment
First you should make sure you have React Query installed in your project:
npm install react-query
Then you’ll need to import the necessary hooks and client from React Query:
import { useQuery, QueryClient, QueryClientProvider } from 'react-query';
How to set up pagination in React Query
To begin with pagination, you need a function to fetch your data. This function typically takes a page number as an argument:
const fetchProjects = async (page = 0) => { const res = await fetch('/api/projects?page=' + page); return res.json(); };
Use the useQuery
hook to fetch data for a specific page:
const Projects = ({ page }) => { const { data, isLoading, isError } = useQuery(['projects', page], () => fetchProjects(page)); // Render your component based on the data };
How to handle page changes
To navigate between pages, you need to manage the page
state and pass it to your query:
const PaginatedProjects = () => { const [page, setPage] = useState(0); return ( <div> <Projects page={page} /> <button onClick={() => setPage(old => Math.max(old - 1, 0))}>Previous Page</button> <button onClick={() => setPage(old => old + 1)}>Next Page</button> </div> ); };
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

How to handle infinite scrolling in react query
If you prefer infinite scrolling over traditional pagination, use useInfiniteQuery
:
const fetchProjectsInfinite = ({ pageParam = 0 }) => { return fetchProjects(pageParam); }; const InfiniteProjects = () => { const { data, fetchNextPage, hasNextPage, isFetchingNextPage, } = useInfiniteQuery('projects', fetchProjectsInfinite, { getNextPageParam: (lastPage, pages) => lastPage.nextPage, }); // Render your component with infinite scroll functionality };
Optimize with React Query’s cache
React Query caches your queries, which means when a user navigates back to a previously visited page, the data is served from the cache, reducing loading times and server requests.
Integrate with external tools
If your project requires an admin panel to view and edit data from your database, consider using tools like Basedash. It allows you to generate admin panels and manage database data efficiently, which can be especially handy when dealing with large datasets in paginated queries.
How to use keepPreviousData
The keepPreviousData
option in React Query is a game-changer for improving user experience in pagination. It allows your application to retain data from a previous page while new data is being loaded. This feature is essential for smoother page transitions and reducing the perceived loading time.
Why you should use keepPreviousData
- Seamless Transitions: By keeping the data of the previous page visible until the new page's data loads, users experience less disruption. This eliminates the jarring effect of a blank screen or a loading spinner during page switches.
- Maintain Scroll Position: Particularly useful in infinite scrolling scenarios, where you want to keep the user's scroll position stable as new data loads.
- Optimized User Experience: It provides a more continuous and fluid browsing experience, which is crucial for keeping users engaged, especially in data-heavy applications.
How to implement keepPreviousData
It’s pretty straightforward to implement. Here's how you can modify the useQuery
hook for paginated data:
const { data, isLoading, isError } = useQuery( ['projects', page], () => fetchProjects(page), { keepPreviousData: true } );
In this example, when the page
state changes (as the user navigates to a different page), the old data (from the previous page) is rendered until the new data is fetched. This usually leads to a better user experience since you’re reducing the abrupt changes that you’ll usually see during data fetching.
Practical Example
Consider a list of projects that users can browse through multiple pages. When a user clicks "Next Page," instead of immediately showing a loading indicator or a blank page, the application continues to show the current page's content. Behind the scenes, the new page's data is being fetched. Once the data is available, the UI updates seamlessly, reducing the cognitive load and disorientation associated with traditional pagination methods.
How to handle loading and error states
Always handle loading and error states in your components:
if (isLoading) return 'Loading...'; if (isError) return 'An error has occurred';
January 29, 2024
Pagination is a common requirement in modern web applications, and React Query offers a straightforward way to implement it. In this guide we’ll walk you through setting up pagination using React Query.
What is pagination in React Query?
Pagination involves splitting a large dataset into smaller, manageable chunks, typically displayed as pages. React Query handles pagination by providing built-in hooks that manage the data fetching and state management aspects. It offers flexibility to implement different types of pagination, like traditional numbered pages or infinite scrolling.
Set up the environment
First you should make sure you have React Query installed in your project:
npm install react-query
Then you’ll need to import the necessary hooks and client from React Query:
import { useQuery, QueryClient, QueryClientProvider } from 'react-query';
How to set up pagination in React Query
To begin with pagination, you need a function to fetch your data. This function typically takes a page number as an argument:
const fetchProjects = async (page = 0) => { const res = await fetch('/api/projects?page=' + page); return res.json(); };
Use the useQuery
hook to fetch data for a specific page:
const Projects = ({ page }) => { const { data, isLoading, isError } = useQuery(['projects', page], () => fetchProjects(page)); // Render your component based on the data };
How to handle page changes
To navigate between pages, you need to manage the page
state and pass it to your query:
const PaginatedProjects = () => { const [page, setPage] = useState(0); return ( <div> <Projects page={page} /> <button onClick={() => setPage(old => Math.max(old - 1, 0))}>Previous Page</button> <button onClick={() => setPage(old => old + 1)}>Next Page</button> </div> ); };
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

How to handle infinite scrolling in react query
If you prefer infinite scrolling over traditional pagination, use useInfiniteQuery
:
const fetchProjectsInfinite = ({ pageParam = 0 }) => { return fetchProjects(pageParam); }; const InfiniteProjects = () => { const { data, fetchNextPage, hasNextPage, isFetchingNextPage, } = useInfiniteQuery('projects', fetchProjectsInfinite, { getNextPageParam: (lastPage, pages) => lastPage.nextPage, }); // Render your component with infinite scroll functionality };
Optimize with React Query’s cache
React Query caches your queries, which means when a user navigates back to a previously visited page, the data is served from the cache, reducing loading times and server requests.
Integrate with external tools
If your project requires an admin panel to view and edit data from your database, consider using tools like Basedash. It allows you to generate admin panels and manage database data efficiently, which can be especially handy when dealing with large datasets in paginated queries.
How to use keepPreviousData
The keepPreviousData
option in React Query is a game-changer for improving user experience in pagination. It allows your application to retain data from a previous page while new data is being loaded. This feature is essential for smoother page transitions and reducing the perceived loading time.
Why you should use keepPreviousData
- Seamless Transitions: By keeping the data of the previous page visible until the new page's data loads, users experience less disruption. This eliminates the jarring effect of a blank screen or a loading spinner during page switches.
- Maintain Scroll Position: Particularly useful in infinite scrolling scenarios, where you want to keep the user's scroll position stable as new data loads.
- Optimized User Experience: It provides a more continuous and fluid browsing experience, which is crucial for keeping users engaged, especially in data-heavy applications.
How to implement keepPreviousData
It’s pretty straightforward to implement. Here's how you can modify the useQuery
hook for paginated data:
const { data, isLoading, isError } = useQuery( ['projects', page], () => fetchProjects(page), { keepPreviousData: true } );
In this example, when the page
state changes (as the user navigates to a different page), the old data (from the previous page) is rendered until the new data is fetched. This usually leads to a better user experience since you’re reducing the abrupt changes that you’ll usually see during data fetching.
Practical Example
Consider a list of projects that users can browse through multiple pages. When a user clicks "Next Page," instead of immediately showing a loading indicator or a blank page, the application continues to show the current page's content. Behind the scenes, the new page's data is being fetched. Once the data is available, the UI updates seamlessly, reducing the cognitive load and disorientation associated with traditional pagination methods.
How to handle loading and error states
Always handle loading and error states in your components:
if (isLoading) return 'Loading...'; if (isError) return 'An error has occurred';
What is Basedash?
What is Basedash?
What is Basedash?
Ship faster, worry less with Basedash
Ship faster, worry less with Basedash
Ship faster, worry less with Basedash
You're busy enough with product work to be weighed down building, maintaining, scoping and developing internal apps and admin panels. Forget all of that, and give your team the admin panel that you don't have to build. Launch in less time than it takes to run a standup.
You're busy enough with product work to be weighed down building, maintaining, scoping and developing internal apps and admin panels. Forget all of that, and give your team the admin panel that you don't have to build. Launch in less time than it takes to run a standup.
You're busy enough with product work to be weighed down building, maintaining, scoping and developing internal apps and admin panels. Forget all of that, and give your team the admin panel that you don't have to build. Launch in less time than it takes to run a standup.




Dashboards and charts
Edit data, create records, oversee how your product is running without the need to build or manage custom software.
USER CRM
ADMIN PANEL
SQL COMPOSER WITH AI

Related posts
Related posts
Related posts



How to Center a Table in HTML with CSS
Jeremy Sarchet



Adjusting HTML Table Column Width for Better Design
Robert Cooper



How to Link Multiple CSS Stylesheets in HTML
Robert Cooper



Mastering HTML Table Inline Styling: A Guide
Max Musing



HTML Multiple Style Attributes: A Quick Guide
Max Musing



How to Set HTML Table Width for Responsive Design
Max Musing