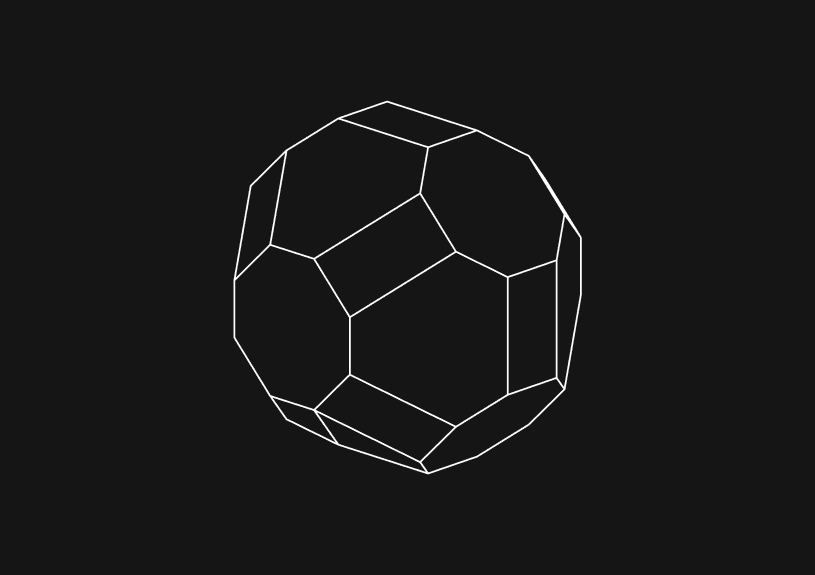
Understanding the “as” keyword in TypeScript
October 23, 2023
In TypeScript, the as
keyword is a type assertion operator. It's a way to tell the TypeScript compiler "trust me, I know what I'm doing." The as
keyword is used to explicitly cast from one type to another. In this guide, we'll explore its usage, nuances, and best practices.
Why use type assertions?
TypeScript's static type checking can prevent many runtime errors. However, sometimes you might be more informed about the type of a particular value than TypeScript's type inference. This is where type assertions come into play.
For instance, when interacting with libraries or APIs that are not strongly typed, or when using legacy JavaScript code, you might need to tell TypeScript what type a particular variable is.
Syntax
The as
keyword provides a way to perform type assertions:
let someValue: any = "this is a string"; let strLength: number = (someValue as string).length;
In the example above, the (someValue as string)
part is asserting that someValue
should be interpreted as a string, even though its type is defined as any
. This allows you to use the length
property without any type errors.
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

Alternatives to as
syntax
TypeScript also supports another, older syntax for type assertions using the angle bracket < >
syntax:
let strLength: number = (<string>someValue).length;
However, the as
syntax is generally recommended, especially when using JSX with React, as the angle bracket syntax can be mistaken for JSX tags.
Key points to remember
- Type assertions do not perform type conversion. They're a way to inform the TypeScript compiler about the type of a variable. At runtime, no type conversion is done.
- Use them sparingly. Over-relying on type assertions can make your code more error-prone. They essentially bypass TypeScript's type checker, so it's like saying, "I know better than the compiler." If you misuse them, you risk introducing runtime errors.
- They don't ensure runtime type safety. Even if you assert a variable is of a certain type, it doesn't mean it'll be that type at runtime. Always ensure data integrity and type safety through runtime checks if uncertain.
Best practices
-
Prefer Type Guards: Instead of type assertions, consider using type guards. They're a way to narrow down the type of an object within a particular scope through type-checking conditions.
if (typeof someValue === 'string') { let strLength = someValue.length; // No assertion needed } -
Be Explicit with
any
: If you're using theany
type and then asserting to a more specific type, it might be a sign you could be more explicit earlier in your code. -
Use with Third-party Libraries: When integrating with JavaScript libraries without TypeScript definitions, type assertions can be helpful. However, it's better to use or create a type definition for the library if possible.
TOC
October 23, 2023
In TypeScript, the as
keyword is a type assertion operator. It's a way to tell the TypeScript compiler "trust me, I know what I'm doing." The as
keyword is used to explicitly cast from one type to another. In this guide, we'll explore its usage, nuances, and best practices.
Why use type assertions?
TypeScript's static type checking can prevent many runtime errors. However, sometimes you might be more informed about the type of a particular value than TypeScript's type inference. This is where type assertions come into play.
For instance, when interacting with libraries or APIs that are not strongly typed, or when using legacy JavaScript code, you might need to tell TypeScript what type a particular variable is.
Syntax
The as
keyword provides a way to perform type assertions:
let someValue: any = "this is a string"; let strLength: number = (someValue as string).length;
In the example above, the (someValue as string)
part is asserting that someValue
should be interpreted as a string, even though its type is defined as any
. This allows you to use the length
property without any type errors.
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

Alternatives to as
syntax
TypeScript also supports another, older syntax for type assertions using the angle bracket < >
syntax:
let strLength: number = (<string>someValue).length;
However, the as
syntax is generally recommended, especially when using JSX with React, as the angle bracket syntax can be mistaken for JSX tags.
Key points to remember
- Type assertions do not perform type conversion. They're a way to inform the TypeScript compiler about the type of a variable. At runtime, no type conversion is done.
- Use them sparingly. Over-relying on type assertions can make your code more error-prone. They essentially bypass TypeScript's type checker, so it's like saying, "I know better than the compiler." If you misuse them, you risk introducing runtime errors.
- They don't ensure runtime type safety. Even if you assert a variable is of a certain type, it doesn't mean it'll be that type at runtime. Always ensure data integrity and type safety through runtime checks if uncertain.
Best practices
-
Prefer Type Guards: Instead of type assertions, consider using type guards. They're a way to narrow down the type of an object within a particular scope through type-checking conditions.
if (typeof someValue === 'string') { let strLength = someValue.length; // No assertion needed } -
Be Explicit with
any
: If you're using theany
type and then asserting to a more specific type, it might be a sign you could be more explicit earlier in your code. -
Use with Third-party Libraries: When integrating with JavaScript libraries without TypeScript definitions, type assertions can be helpful. However, it's better to use or create a type definition for the library if possible.
October 23, 2023
In TypeScript, the as
keyword is a type assertion operator. It's a way to tell the TypeScript compiler "trust me, I know what I'm doing." The as
keyword is used to explicitly cast from one type to another. In this guide, we'll explore its usage, nuances, and best practices.
Why use type assertions?
TypeScript's static type checking can prevent many runtime errors. However, sometimes you might be more informed about the type of a particular value than TypeScript's type inference. This is where type assertions come into play.
For instance, when interacting with libraries or APIs that are not strongly typed, or when using legacy JavaScript code, you might need to tell TypeScript what type a particular variable is.
Syntax
The as
keyword provides a way to perform type assertions:
let someValue: any = "this is a string"; let strLength: number = (someValue as string).length;
In the example above, the (someValue as string)
part is asserting that someValue
should be interpreted as a string, even though its type is defined as any
. This allows you to use the length
property without any type errors.
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

Alternatives to as
syntax
TypeScript also supports another, older syntax for type assertions using the angle bracket < >
syntax:
let strLength: number = (<string>someValue).length;
However, the as
syntax is generally recommended, especially when using JSX with React, as the angle bracket syntax can be mistaken for JSX tags.
Key points to remember
- Type assertions do not perform type conversion. They're a way to inform the TypeScript compiler about the type of a variable. At runtime, no type conversion is done.
- Use them sparingly. Over-relying on type assertions can make your code more error-prone. They essentially bypass TypeScript's type checker, so it's like saying, "I know better than the compiler." If you misuse them, you risk introducing runtime errors.
- They don't ensure runtime type safety. Even if you assert a variable is of a certain type, it doesn't mean it'll be that type at runtime. Always ensure data integrity and type safety through runtime checks if uncertain.
Best practices
-
Prefer Type Guards: Instead of type assertions, consider using type guards. They're a way to narrow down the type of an object within a particular scope through type-checking conditions.
if (typeof someValue === 'string') { let strLength = someValue.length; // No assertion needed } -
Be Explicit with
any
: If you're using theany
type and then asserting to a more specific type, it might be a sign you could be more explicit earlier in your code. -
Use with Third-party Libraries: When integrating with JavaScript libraries without TypeScript definitions, type assertions can be helpful. However, it's better to use or create a type definition for the library if possible.
What is Basedash?
What is Basedash?
What is Basedash?
Ship faster, worry less with Basedash
Ship faster, worry less with Basedash
You're busy enough with product work to be weighed down building, maintaining, scoping and developing internal apps and admin panels. Forget all of that, and give your team the admin panel that you don't have to build. Launch in less time than it takes to run a standup.
You're busy enough with product work to be weighed down building, maintaining, scoping and developing internal apps and admin panels. Forget all of that, and give your team the admin panel that you don't have to build. Launch in less time than it takes to run a standup.
You're busy enough with product work to be weighed down building, maintaining, scoping and developing internal apps and admin panels. Forget all of that, and give your team the admin panel that you don't have to build. Launch in less time than it takes to run a standup.




Dashboards and charts
Edit data, create records, oversee how your product is running without the need to build or manage custom software.
USER CRM
ADMIN PANEL
SQL COMPOSER WITH AI

Related posts
Related posts
Related posts



How to turn webpages into editable canvases with a JavaScript bookmarklet
Kris Lachance



How to fix the "not all code paths return a value" issue in TypeScript
Kris Lachance



Working with WebSockets in Node.js using TypeScript
Kris Lachance



Type Annotations Can Only Be Used in TypeScript Files
Kris Lachance



Guide to TypeScript Recursive Type
Kris Lachance



How to Configure Knex.js with TypeScript
Kris Lachance