
How to compare dates in JavaScript
November 10, 2023
You’ll often have to compare two dates in JavaScript, usually to schedule events, log activities or determine time differences. This guide will take you through various methods to compare dates, including how to ignore the time component for pure date comparisons.
Can you compare dates in JavaScript?
Yes! You can do this by creating a Date
object. We’ll cover this below.
What are date objects in JavaScript?
JS has a built-in Date
object that represents a single moment in time. Date objects are based on a time value that is the number of milliseconds since 1 January 1970 UTC.
To create a new date object for the current time, you would write:
let currentDate = new Date();
To compare 2 dates, you need to understand that JS allows date objects to be compared using comparison operators.
Compare two dates with time
When you're comparing two dates with time, you're interested in the precise moment they represent.
let date1 = new Date('2023-01-01T00:00:00'); let date2 = new Date('2023-12-31T23:59:59'); // Comparing dates if (date1 > date2) { console.log('Date1 is later than Date2'); } else if (date1 < date2) { console.log('Date1 is earlier than Date2'); } else { console.log('Date1 is the same as Date2'); }
The >
and <
operators check if one date is before or after another, while ==
checks if they are the exact same moment in time.
How to compare dates without time in JavaScript
Sometimes, you may need to compare the dates without considering the time. This involves setting the time to the same value on both dates or extracting the date components to compare.
Setting time to 00:00:00
let date1 = new Date('2023-01-01'); let date2 = new Date('2023-12-31'); // Reset the time part to ensure only date comparison date1.setHours(0, 0, 0, 0); date2.setHours(0, 0, 0, 0); if (date1 > date2) { console.log('Date1 is later than Date2'); } else if (date1 < date2) { console.log('Date1 is earlier than Date2'); } else { console.log('Date1 is the same as Date2'); }
By setting the hours, minutes, seconds, and milliseconds to zero, you ensure that only the date part affects the comparison.
Compare date components
Alternatively, you can compare the year, month, and day values individually.
let date1 = new Date('2023-01-01'); let date2 = new Date('2023-12-31'); if (date1.getFullYear() === date2.getFullYear() && date1.getMonth() === date2.getMonth() && date1.getDate() === date2.getDate()) { console.log('Date1 is the same as Date2'); } else { console.log('Date1 and Date2 are different dates'); }
This method allows you to directly compare the date-related components without modifying the time component of the Date
objects.
Using third-party libraries
For complex date comparisons, you might opt for libraries like moment.js
or date-fns
, which offer a more fluent and error-proof API.
Moment.js example
const moment = require('moment'); let date1 = moment('2023-01-01'); let date2 = moment('2023-12-31'); if (date1.isSame(date2, 'day')) { console.log('Date1 is the same as Date2'); } else if (date1.isAfter(date2)) { console.log('Date1 is later than Date2'); } else { console.log('Date1 is earlier than Date2'); }
moment.js
provides the isSame
, isAfter
, and isBefore
methods which can be used to compare dates with ease.
Date-fns example
const dateFns = require('date-fns'); let date1 = new Date('2023-01-01'); let date2 = new Date('2023-12-31'); if (dateFns.isSameDay(date1, date2)) { console.log('Date1 is the same as Date2'); } else if (dateFns.isAfter(date1, date2)) { console.log('Date1 is later than Date2'); } else { console.log('Date1 is earlier than Date2'); }
date-fns
is a modular library, and you can import only the functions you need, such as isSameDay
, isAfter
, and isBefore
.
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

How to deal with timezones
When comparing two dates, especially in web applications, you may need to account for timezones.
let date1 = new Date('2023-01-01T00:00:00Z'); // UTC time let date2 = new Date('2023-01-01T00:00:00-05:00'); // Eastern Standard Time date1.setHours(0, 0, 0, 0); date2.setHours(0, 0, 0, 0); if (date1.toISOString() === date2.toISOString()) { console.log('Date1 is the same as Date2 in UTC'); }
Using toISOString()
normalizes both dates to UTC, allowing for a fair comparison.
Handling edge cases in date comparisons
Watch out for special cases such as leap seconds, leap years, and daylight saving time:
let leapDay = new Date('2024-02-29'); let standardDay = new Date('2023-03-01'); if (leapDay < standardDay) { console.log('Leap day comes before the standard day'); }
How to deal with multiple locales
When dealing with multiple locales, use the user's locale to parse and compare dates accurately:
let usDate = new Date('07/04/2023'); let euDate = new Date('04/07/2023'); let dateFormatter = new Intl.DateTimeFormat('en-US'); let usFormattedDate = dateFormatter.format(usDate); let euFormattedDate = dateFormatter.format(euDate); console.log(usFormattedDate === euFormattedDate); // Locale affects this result
How to deal with invalid dates
Before comparing, ensure that you're not dealing with an invalid date, which can lead to misleading comparisons:
let invalidDate = new Date('not a real date'); if (isNaN(invalidDate.getTime())) { console.log('This is an invalid date'); }
Conclusion
Comparing dates in JavaScript can range from a simple direct comparison using the Date
object's inherent capabilities to more complex scenarios where libraries like moment.js
and date-fns
can be quite helpful. When working with dates, always consider the implications of time zones and daylight saving changes. Whether you need to compare the exact time or just the date component, JavaScript provides several methods to achieve accurate results.
FAQs
Can you compare two dates in JavaScript?
Yes! You can most commonly do this by creating a Date
object.
When comparing Date objects, you're essentially comparing the timestamps (milliseconds since the Unix epoch).
If you want to explicitly work with the timestamp values, you can use the getTime()
method.
What is the best way to compare two dates in JavaScript?
If you're looking to find out if one date is before, after, or the same as another, you can directly use comparison operators with Date
objects.
How to compare two dates in DD MMM YYYY format in JavaScript?
You can compare two dates in DD MMM YYYY format in JavaScript by first converting them to Date objects and then using the standard comparison operators (>, <, ==, etc.).
How to compare 3 dates in JavaScript?
In JavaScript, you can compare dates by first creating Date
objects and then comparing them using the usual comparison operators (<
, >
, <=
, >=
, ==
, !=
).
Can you compare two dates as strings?
Yes, in JavaScript, you can compare two date strings directly, as long as they are in a format that the Date
constructor can parse, such as the ISO 8601 format (YYYY-MM-DD
or YYYY-MM-DDTHH:MM:SSZ
). When you create Date
objects from these strings, JavaScript automatically converts them into date objects that represent a specific moment in time, and you can then compare these objects.
How to compare two dates and months in JavaScript?
To compare just the months and days without considering the year, you would have to get the month and day from the date objects and then compare them.
How to check if two dates fall on the exact same day in JavaScript?
In JavaScript, you can check if two dates fall on the exact same day by comparing the day, month, and year values of each date object.
How to get the second difference between two dates in JavaScript?
In JavaScript, you can get the difference between two dates in milliseconds by subtracting two date objects. Once you have the difference in milliseconds, you can convert it to seconds by dividing by 1000. To get the second difference, you would simply need to round to the nearest second if you want an integer value.
TOC
November 10, 2023
You’ll often have to compare two dates in JavaScript, usually to schedule events, log activities or determine time differences. This guide will take you through various methods to compare dates, including how to ignore the time component for pure date comparisons.
Can you compare dates in JavaScript?
Yes! You can do this by creating a Date
object. We’ll cover this below.
What are date objects in JavaScript?
JS has a built-in Date
object that represents a single moment in time. Date objects are based on a time value that is the number of milliseconds since 1 January 1970 UTC.
To create a new date object for the current time, you would write:
let currentDate = new Date();
To compare 2 dates, you need to understand that JS allows date objects to be compared using comparison operators.
Compare two dates with time
When you're comparing two dates with time, you're interested in the precise moment they represent.
let date1 = new Date('2023-01-01T00:00:00'); let date2 = new Date('2023-12-31T23:59:59'); // Comparing dates if (date1 > date2) { console.log('Date1 is later than Date2'); } else if (date1 < date2) { console.log('Date1 is earlier than Date2'); } else { console.log('Date1 is the same as Date2'); }
The >
and <
operators check if one date is before or after another, while ==
checks if they are the exact same moment in time.
How to compare dates without time in JavaScript
Sometimes, you may need to compare the dates without considering the time. This involves setting the time to the same value on both dates or extracting the date components to compare.
Setting time to 00:00:00
let date1 = new Date('2023-01-01'); let date2 = new Date('2023-12-31'); // Reset the time part to ensure only date comparison date1.setHours(0, 0, 0, 0); date2.setHours(0, 0, 0, 0); if (date1 > date2) { console.log('Date1 is later than Date2'); } else if (date1 < date2) { console.log('Date1 is earlier than Date2'); } else { console.log('Date1 is the same as Date2'); }
By setting the hours, minutes, seconds, and milliseconds to zero, you ensure that only the date part affects the comparison.
Compare date components
Alternatively, you can compare the year, month, and day values individually.
let date1 = new Date('2023-01-01'); let date2 = new Date('2023-12-31'); if (date1.getFullYear() === date2.getFullYear() && date1.getMonth() === date2.getMonth() && date1.getDate() === date2.getDate()) { console.log('Date1 is the same as Date2'); } else { console.log('Date1 and Date2 are different dates'); }
This method allows you to directly compare the date-related components without modifying the time component of the Date
objects.
Using third-party libraries
For complex date comparisons, you might opt for libraries like moment.js
or date-fns
, which offer a more fluent and error-proof API.
Moment.js example
const moment = require('moment'); let date1 = moment('2023-01-01'); let date2 = moment('2023-12-31'); if (date1.isSame(date2, 'day')) { console.log('Date1 is the same as Date2'); } else if (date1.isAfter(date2)) { console.log('Date1 is later than Date2'); } else { console.log('Date1 is earlier than Date2'); }
moment.js
provides the isSame
, isAfter
, and isBefore
methods which can be used to compare dates with ease.
Date-fns example
const dateFns = require('date-fns'); let date1 = new Date('2023-01-01'); let date2 = new Date('2023-12-31'); if (dateFns.isSameDay(date1, date2)) { console.log('Date1 is the same as Date2'); } else if (dateFns.isAfter(date1, date2)) { console.log('Date1 is later than Date2'); } else { console.log('Date1 is earlier than Date2'); }
date-fns
is a modular library, and you can import only the functions you need, such as isSameDay
, isAfter
, and isBefore
.
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

How to deal with timezones
When comparing two dates, especially in web applications, you may need to account for timezones.
let date1 = new Date('2023-01-01T00:00:00Z'); // UTC time let date2 = new Date('2023-01-01T00:00:00-05:00'); // Eastern Standard Time date1.setHours(0, 0, 0, 0); date2.setHours(0, 0, 0, 0); if (date1.toISOString() === date2.toISOString()) { console.log('Date1 is the same as Date2 in UTC'); }
Using toISOString()
normalizes both dates to UTC, allowing for a fair comparison.
Handling edge cases in date comparisons
Watch out for special cases such as leap seconds, leap years, and daylight saving time:
let leapDay = new Date('2024-02-29'); let standardDay = new Date('2023-03-01'); if (leapDay < standardDay) { console.log('Leap day comes before the standard day'); }
How to deal with multiple locales
When dealing with multiple locales, use the user's locale to parse and compare dates accurately:
let usDate = new Date('07/04/2023'); let euDate = new Date('04/07/2023'); let dateFormatter = new Intl.DateTimeFormat('en-US'); let usFormattedDate = dateFormatter.format(usDate); let euFormattedDate = dateFormatter.format(euDate); console.log(usFormattedDate === euFormattedDate); // Locale affects this result
How to deal with invalid dates
Before comparing, ensure that you're not dealing with an invalid date, which can lead to misleading comparisons:
let invalidDate = new Date('not a real date'); if (isNaN(invalidDate.getTime())) { console.log('This is an invalid date'); }
Conclusion
Comparing dates in JavaScript can range from a simple direct comparison using the Date
object's inherent capabilities to more complex scenarios where libraries like moment.js
and date-fns
can be quite helpful. When working with dates, always consider the implications of time zones and daylight saving changes. Whether you need to compare the exact time or just the date component, JavaScript provides several methods to achieve accurate results.
FAQs
Can you compare two dates in JavaScript?
Yes! You can most commonly do this by creating a Date
object.
When comparing Date objects, you're essentially comparing the timestamps (milliseconds since the Unix epoch).
If you want to explicitly work with the timestamp values, you can use the getTime()
method.
What is the best way to compare two dates in JavaScript?
If you're looking to find out if one date is before, after, or the same as another, you can directly use comparison operators with Date
objects.
How to compare two dates in DD MMM YYYY format in JavaScript?
You can compare two dates in DD MMM YYYY format in JavaScript by first converting them to Date objects and then using the standard comparison operators (>, <, ==, etc.).
How to compare 3 dates in JavaScript?
In JavaScript, you can compare dates by first creating Date
objects and then comparing them using the usual comparison operators (<
, >
, <=
, >=
, ==
, !=
).
Can you compare two dates as strings?
Yes, in JavaScript, you can compare two date strings directly, as long as they are in a format that the Date
constructor can parse, such as the ISO 8601 format (YYYY-MM-DD
or YYYY-MM-DDTHH:MM:SSZ
). When you create Date
objects from these strings, JavaScript automatically converts them into date objects that represent a specific moment in time, and you can then compare these objects.
How to compare two dates and months in JavaScript?
To compare just the months and days without considering the year, you would have to get the month and day from the date objects and then compare them.
How to check if two dates fall on the exact same day in JavaScript?
In JavaScript, you can check if two dates fall on the exact same day by comparing the day, month, and year values of each date object.
How to get the second difference between two dates in JavaScript?
In JavaScript, you can get the difference between two dates in milliseconds by subtracting two date objects. Once you have the difference in milliseconds, you can convert it to seconds by dividing by 1000. To get the second difference, you would simply need to round to the nearest second if you want an integer value.
November 10, 2023
You’ll often have to compare two dates in JavaScript, usually to schedule events, log activities or determine time differences. This guide will take you through various methods to compare dates, including how to ignore the time component for pure date comparisons.
Can you compare dates in JavaScript?
Yes! You can do this by creating a Date
object. We’ll cover this below.
What are date objects in JavaScript?
JS has a built-in Date
object that represents a single moment in time. Date objects are based on a time value that is the number of milliseconds since 1 January 1970 UTC.
To create a new date object for the current time, you would write:
let currentDate = new Date();
To compare 2 dates, you need to understand that JS allows date objects to be compared using comparison operators.
Compare two dates with time
When you're comparing two dates with time, you're interested in the precise moment they represent.
let date1 = new Date('2023-01-01T00:00:00'); let date2 = new Date('2023-12-31T23:59:59'); // Comparing dates if (date1 > date2) { console.log('Date1 is later than Date2'); } else if (date1 < date2) { console.log('Date1 is earlier than Date2'); } else { console.log('Date1 is the same as Date2'); }
The >
and <
operators check if one date is before or after another, while ==
checks if they are the exact same moment in time.
How to compare dates without time in JavaScript
Sometimes, you may need to compare the dates without considering the time. This involves setting the time to the same value on both dates or extracting the date components to compare.
Setting time to 00:00:00
let date1 = new Date('2023-01-01'); let date2 = new Date('2023-12-31'); // Reset the time part to ensure only date comparison date1.setHours(0, 0, 0, 0); date2.setHours(0, 0, 0, 0); if (date1 > date2) { console.log('Date1 is later than Date2'); } else if (date1 < date2) { console.log('Date1 is earlier than Date2'); } else { console.log('Date1 is the same as Date2'); }
By setting the hours, minutes, seconds, and milliseconds to zero, you ensure that only the date part affects the comparison.
Compare date components
Alternatively, you can compare the year, month, and day values individually.
let date1 = new Date('2023-01-01'); let date2 = new Date('2023-12-31'); if (date1.getFullYear() === date2.getFullYear() && date1.getMonth() === date2.getMonth() && date1.getDate() === date2.getDate()) { console.log('Date1 is the same as Date2'); } else { console.log('Date1 and Date2 are different dates'); }
This method allows you to directly compare the date-related components without modifying the time component of the Date
objects.
Using third-party libraries
For complex date comparisons, you might opt for libraries like moment.js
or date-fns
, which offer a more fluent and error-proof API.
Moment.js example
const moment = require('moment'); let date1 = moment('2023-01-01'); let date2 = moment('2023-12-31'); if (date1.isSame(date2, 'day')) { console.log('Date1 is the same as Date2'); } else if (date1.isAfter(date2)) { console.log('Date1 is later than Date2'); } else { console.log('Date1 is earlier than Date2'); }
moment.js
provides the isSame
, isAfter
, and isBefore
methods which can be used to compare dates with ease.
Date-fns example
const dateFns = require('date-fns'); let date1 = new Date('2023-01-01'); let date2 = new Date('2023-12-31'); if (dateFns.isSameDay(date1, date2)) { console.log('Date1 is the same as Date2'); } else if (dateFns.isAfter(date1, date2)) { console.log('Date1 is later than Date2'); } else { console.log('Date1 is earlier than Date2'); }
date-fns
is a modular library, and you can import only the functions you need, such as isSameDay
, isAfter
, and isBefore
.
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

How to deal with timezones
When comparing two dates, especially in web applications, you may need to account for timezones.
let date1 = new Date('2023-01-01T00:00:00Z'); // UTC time let date2 = new Date('2023-01-01T00:00:00-05:00'); // Eastern Standard Time date1.setHours(0, 0, 0, 0); date2.setHours(0, 0, 0, 0); if (date1.toISOString() === date2.toISOString()) { console.log('Date1 is the same as Date2 in UTC'); }
Using toISOString()
normalizes both dates to UTC, allowing for a fair comparison.
Handling edge cases in date comparisons
Watch out for special cases such as leap seconds, leap years, and daylight saving time:
let leapDay = new Date('2024-02-29'); let standardDay = new Date('2023-03-01'); if (leapDay < standardDay) { console.log('Leap day comes before the standard day'); }
How to deal with multiple locales
When dealing with multiple locales, use the user's locale to parse and compare dates accurately:
let usDate = new Date('07/04/2023'); let euDate = new Date('04/07/2023'); let dateFormatter = new Intl.DateTimeFormat('en-US'); let usFormattedDate = dateFormatter.format(usDate); let euFormattedDate = dateFormatter.format(euDate); console.log(usFormattedDate === euFormattedDate); // Locale affects this result
How to deal with invalid dates
Before comparing, ensure that you're not dealing with an invalid date, which can lead to misleading comparisons:
let invalidDate = new Date('not a real date'); if (isNaN(invalidDate.getTime())) { console.log('This is an invalid date'); }
Conclusion
Comparing dates in JavaScript can range from a simple direct comparison using the Date
object's inherent capabilities to more complex scenarios where libraries like moment.js
and date-fns
can be quite helpful. When working with dates, always consider the implications of time zones and daylight saving changes. Whether you need to compare the exact time or just the date component, JavaScript provides several methods to achieve accurate results.
FAQs
Can you compare two dates in JavaScript?
Yes! You can most commonly do this by creating a Date
object.
When comparing Date objects, you're essentially comparing the timestamps (milliseconds since the Unix epoch).
If you want to explicitly work with the timestamp values, you can use the getTime()
method.
What is the best way to compare two dates in JavaScript?
If you're looking to find out if one date is before, after, or the same as another, you can directly use comparison operators with Date
objects.
How to compare two dates in DD MMM YYYY format in JavaScript?
You can compare two dates in DD MMM YYYY format in JavaScript by first converting them to Date objects and then using the standard comparison operators (>, <, ==, etc.).
How to compare 3 dates in JavaScript?
In JavaScript, you can compare dates by first creating Date
objects and then comparing them using the usual comparison operators (<
, >
, <=
, >=
, ==
, !=
).
Can you compare two dates as strings?
Yes, in JavaScript, you can compare two date strings directly, as long as they are in a format that the Date
constructor can parse, such as the ISO 8601 format (YYYY-MM-DD
or YYYY-MM-DDTHH:MM:SSZ
). When you create Date
objects from these strings, JavaScript automatically converts them into date objects that represent a specific moment in time, and you can then compare these objects.
How to compare two dates and months in JavaScript?
To compare just the months and days without considering the year, you would have to get the month and day from the date objects and then compare them.
How to check if two dates fall on the exact same day in JavaScript?
In JavaScript, you can check if two dates fall on the exact same day by comparing the day, month, and year values of each date object.
How to get the second difference between two dates in JavaScript?
In JavaScript, you can get the difference between two dates in milliseconds by subtracting two date objects. Once you have the difference in milliseconds, you can convert it to seconds by dividing by 1000. To get the second difference, you would simply need to round to the nearest second if you want an integer value.
What is Basedash?
What is Basedash?
What is Basedash?
Basedash is the best MySQL admin panel
Basedash is the best MySQL admin panel
Basedash is the best MySQL admin panel
If you're building with MySQL, you need Basedash. It gives you an instantly generated admin panel to understand, query, build dashboards, edit, and share access to your data.
If you're building with MySQL, you need Basedash. It gives you an instantly generated admin panel to understand, query, build dashboards, edit, and share access to your data.
If you're building with MySQL, you need Basedash. It gives you an instantly generated admin panel to understand, query, build dashboards, edit, and share access to your data.




Dashboards and charts
Edit data, create records, oversee how your product is running without the need to build or manage custom software.
USER CRM
ADMIN PANEL
SQL COMPOSER WITH AI

Related posts
Related posts
Related posts



How to Add Columns to MySQL Tables with ALTER TABLE
Robert Cooper



How to Add Columns to Your MySQL Table
Max Musing



Pivot Tables in MySQL
Robert Cooper



How to Rename a Table in MySQL
Max Musing
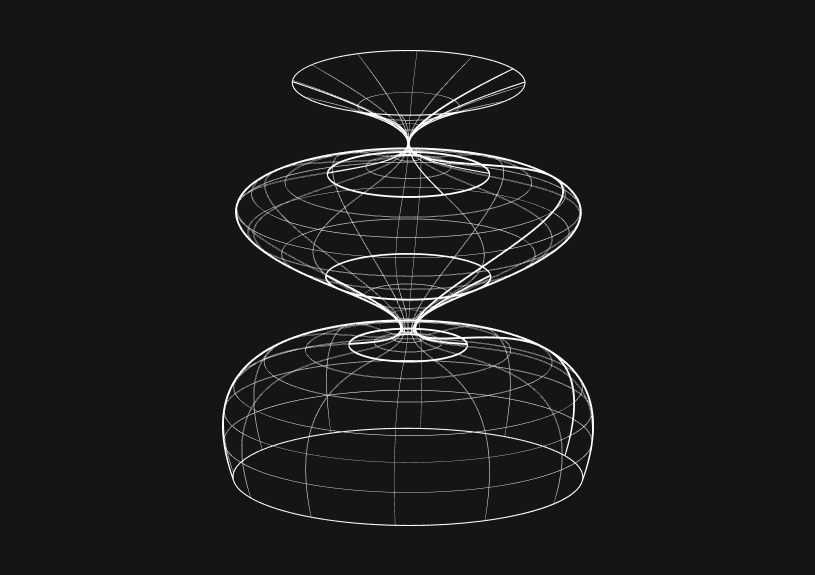
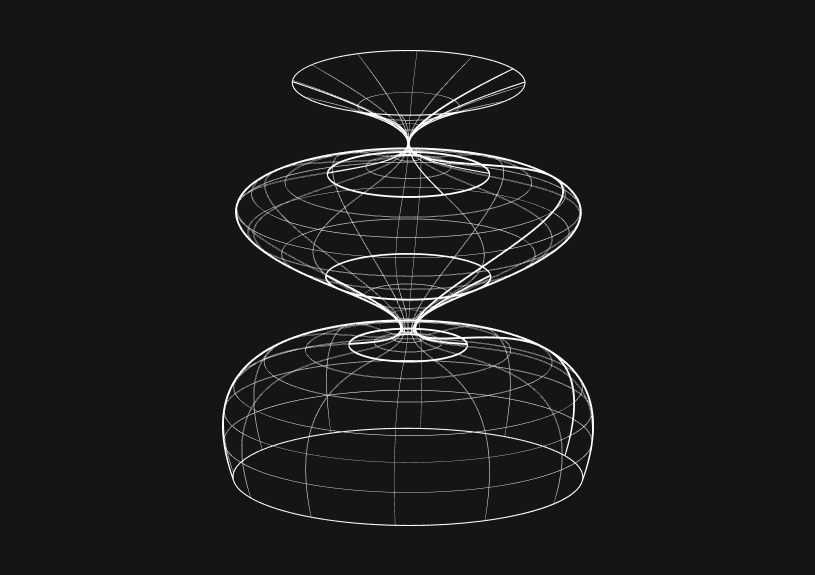
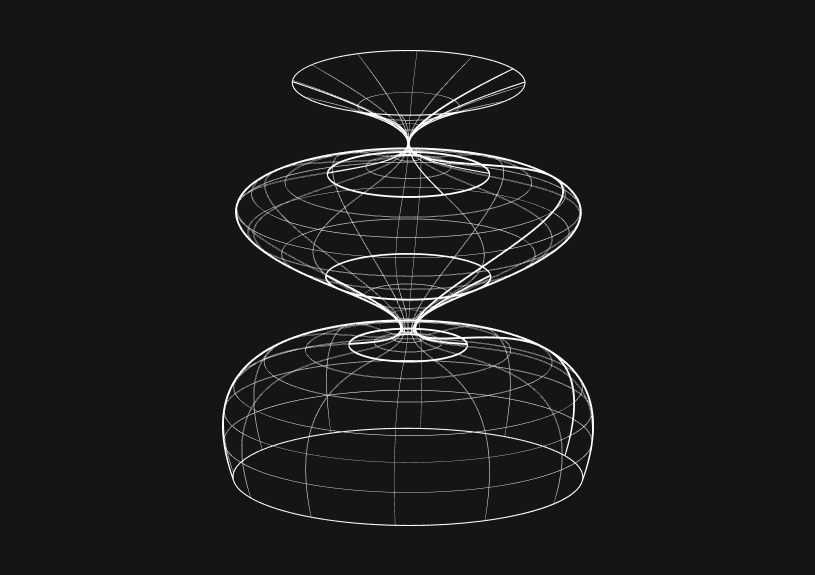
How to Optimize MySQL Tables for Better Performance
Robert Cooper



How to Display MySQL Table Schema: A Guide
Jeremy Sarchet