
How to Debug MySQL Stored Procedures
December 1, 2023
Debugging MySQL stored procedures is tricky, mostly because MySQL’s native debugging tools have some limitations. We’ll cover how to get around them in this guide.
Understanding the procedure's behavior
Start by thoroughly understanding what the stored procedure is supposed to do. Review the procedure’s logic and expected outcomes for various inputs. Use comments within the code to document the procedure's flow and logic.
DELIMITER // CREATE PROCEDURE SampleProcedure() BEGIN -- Procedure logic goes here END // DELIMITER ;
Implementing debugging echoes
One simple debugging technique is to insert SELECT
statements at strategic points in your procedure. These statements can output variable values or indicate the execution flow, helping you track the procedure's behavior.
BEGIN DECLARE debug_variable INT; -- Debugging echo SELECT 'Procedure started' AS debug_info; -- Procedure logic SET debug_variable = 1; SELECT debug_variable AS debug_value; END
Use conditional logging
Incorporate conditional logging in your procedures. This involves creating a debug table and inserting log entries based on a debug flag. This allows you to enable or disable logging as needed.
CREATE TABLE debug_log ( log_id INT AUTO_INCREMENT PRIMARY KEY, message VARCHAR(255), created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP ); BEGIN DECLARE debug_mode BOOLEAN DEFAULT FALSE; IF debug_mode THEN INSERT INTO debug_log(message) VALUES ('Debug message'); END IF; -- Procedure logic END
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

Analyze execution flow
To understand the flow of execution within your stored procedure, especially in loops or conditional statements, use SELECT
statements or conditional logging to indicate which branches of code are being executed.
BEGIN IF condition THEN -- Debugging echo SELECT 'Condition met' AS debug_info; -- Code for condition ELSE -- Debugging echo SELECT 'Condition not met' AS debug_info; -- Alternative code END IF; END
Error handling with try-catch
MySQL doesn't support try-catch blocks like some other SQL databases, but you can simulate it using condition handlers. This allows you to catch and handle errors within your stored procedures.
BEGIN DECLARE EXIT HANDLER FOR SQLEXCEPTION BEGIN -- Error handling code END; -- Procedure logic END
Testing with different data sets
Test your stored procedure with a variety of data sets, including edge cases. This can help uncover issues that may not be apparent with typical data.
Using external tools
Consider using external tools for more advanced debugging. Tools like MySQL Workbench offer features for debugging stored procedures, although they might require additional setup.
TOC
December 1, 2023
Debugging MySQL stored procedures is tricky, mostly because MySQL’s native debugging tools have some limitations. We’ll cover how to get around them in this guide.
Understanding the procedure's behavior
Start by thoroughly understanding what the stored procedure is supposed to do. Review the procedure’s logic and expected outcomes for various inputs. Use comments within the code to document the procedure's flow and logic.
DELIMITER // CREATE PROCEDURE SampleProcedure() BEGIN -- Procedure logic goes here END // DELIMITER ;
Implementing debugging echoes
One simple debugging technique is to insert SELECT
statements at strategic points in your procedure. These statements can output variable values or indicate the execution flow, helping you track the procedure's behavior.
BEGIN DECLARE debug_variable INT; -- Debugging echo SELECT 'Procedure started' AS debug_info; -- Procedure logic SET debug_variable = 1; SELECT debug_variable AS debug_value; END
Use conditional logging
Incorporate conditional logging in your procedures. This involves creating a debug table and inserting log entries based on a debug flag. This allows you to enable or disable logging as needed.
CREATE TABLE debug_log ( log_id INT AUTO_INCREMENT PRIMARY KEY, message VARCHAR(255), created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP ); BEGIN DECLARE debug_mode BOOLEAN DEFAULT FALSE; IF debug_mode THEN INSERT INTO debug_log(message) VALUES ('Debug message'); END IF; -- Procedure logic END
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

Analyze execution flow
To understand the flow of execution within your stored procedure, especially in loops or conditional statements, use SELECT
statements or conditional logging to indicate which branches of code are being executed.
BEGIN IF condition THEN -- Debugging echo SELECT 'Condition met' AS debug_info; -- Code for condition ELSE -- Debugging echo SELECT 'Condition not met' AS debug_info; -- Alternative code END IF; END
Error handling with try-catch
MySQL doesn't support try-catch blocks like some other SQL databases, but you can simulate it using condition handlers. This allows you to catch and handle errors within your stored procedures.
BEGIN DECLARE EXIT HANDLER FOR SQLEXCEPTION BEGIN -- Error handling code END; -- Procedure logic END
Testing with different data sets
Test your stored procedure with a variety of data sets, including edge cases. This can help uncover issues that may not be apparent with typical data.
Using external tools
Consider using external tools for more advanced debugging. Tools like MySQL Workbench offer features for debugging stored procedures, although they might require additional setup.
December 1, 2023
Debugging MySQL stored procedures is tricky, mostly because MySQL’s native debugging tools have some limitations. We’ll cover how to get around them in this guide.
Understanding the procedure's behavior
Start by thoroughly understanding what the stored procedure is supposed to do. Review the procedure’s logic and expected outcomes for various inputs. Use comments within the code to document the procedure's flow and logic.
DELIMITER // CREATE PROCEDURE SampleProcedure() BEGIN -- Procedure logic goes here END // DELIMITER ;
Implementing debugging echoes
One simple debugging technique is to insert SELECT
statements at strategic points in your procedure. These statements can output variable values or indicate the execution flow, helping you track the procedure's behavior.
BEGIN DECLARE debug_variable INT; -- Debugging echo SELECT 'Procedure started' AS debug_info; -- Procedure logic SET debug_variable = 1; SELECT debug_variable AS debug_value; END
Use conditional logging
Incorporate conditional logging in your procedures. This involves creating a debug table and inserting log entries based on a debug flag. This allows you to enable or disable logging as needed.
CREATE TABLE debug_log ( log_id INT AUTO_INCREMENT PRIMARY KEY, message VARCHAR(255), created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP ); BEGIN DECLARE debug_mode BOOLEAN DEFAULT FALSE; IF debug_mode THEN INSERT INTO debug_log(message) VALUES ('Debug message'); END IF; -- Procedure logic END
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

Analyze execution flow
To understand the flow of execution within your stored procedure, especially in loops or conditional statements, use SELECT
statements or conditional logging to indicate which branches of code are being executed.
BEGIN IF condition THEN -- Debugging echo SELECT 'Condition met' AS debug_info; -- Code for condition ELSE -- Debugging echo SELECT 'Condition not met' AS debug_info; -- Alternative code END IF; END
Error handling with try-catch
MySQL doesn't support try-catch blocks like some other SQL databases, but you can simulate it using condition handlers. This allows you to catch and handle errors within your stored procedures.
BEGIN DECLARE EXIT HANDLER FOR SQLEXCEPTION BEGIN -- Error handling code END; -- Procedure logic END
Testing with different data sets
Test your stored procedure with a variety of data sets, including edge cases. This can help uncover issues that may not be apparent with typical data.
Using external tools
Consider using external tools for more advanced debugging. Tools like MySQL Workbench offer features for debugging stored procedures, although they might require additional setup.
What is Basedash?
What is Basedash?
What is Basedash?
Basedash is the best MySQL admin panel
Basedash is the best MySQL admin panel
Basedash is the best MySQL admin panel
If you're building with MySQL, you need Basedash. It gives you an instantly generated admin panel to understand, query, build dashboards, edit, and share access to your data.
If you're building with MySQL, you need Basedash. It gives you an instantly generated admin panel to understand, query, build dashboards, edit, and share access to your data.
If you're building with MySQL, you need Basedash. It gives you an instantly generated admin panel to understand, query, build dashboards, edit, and share access to your data.




Dashboards and charts
Edit data, create records, oversee how your product is running without the need to build or manage custom software.
USER CRM
ADMIN PANEL
SQL COMPOSER WITH AI

Related posts
Related posts
Related posts



How to Add Columns to MySQL Tables with ALTER TABLE
Robert Cooper



How to Add Columns to Your MySQL Table
Max Musing



Pivot Tables in MySQL
Robert Cooper



How to Rename a Table in MySQL
Max Musing
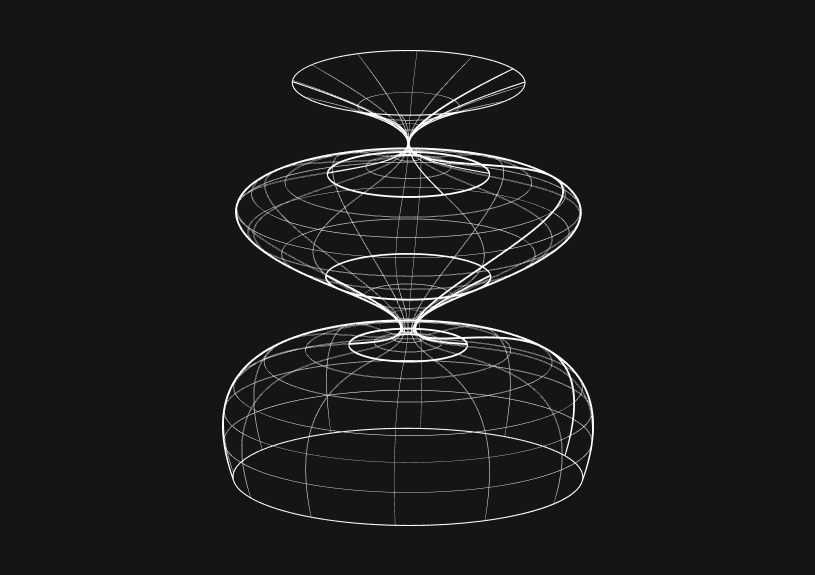
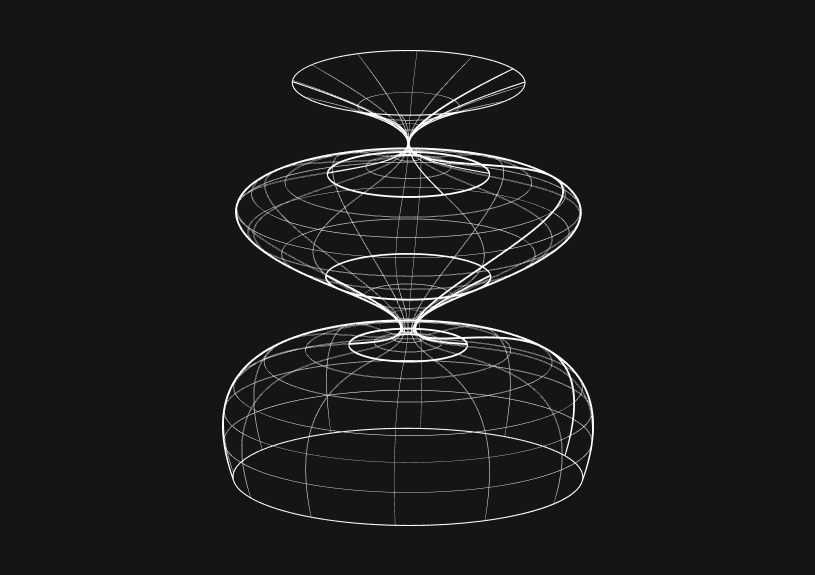
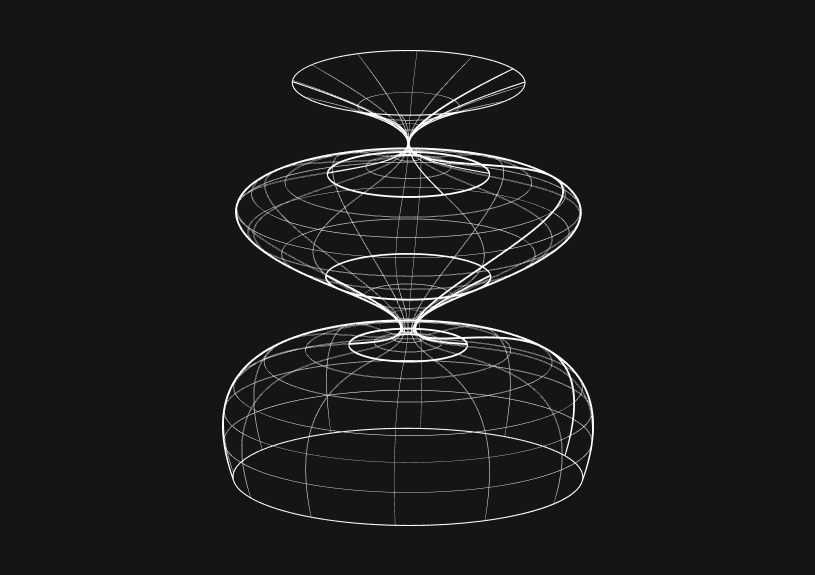
How to Optimize MySQL Tables for Better Performance
Robert Cooper



How to Display MySQL Table Schema: A Guide
Jeremy Sarchet