
MySQL Query Parameters
January 4, 2024
MySQL query parameters are placeholders in SQL statements that are replaced with actual values during execution. They enhance security by preventing SQL injection attacks and improve query efficiency by allowing the database to cache prepared statements.
Understanding Query Parameters
Query parameters in MySQL are used in prepared statements. A prepared statement is a feature used to execute the same statement repeatedly with high efficiency. Parameters in these statements act as placeholders for actual values that are substituted in at execution time.
Example of a Query Parameter
PREPARE stmt FROM 'SELECT * FROM users WHERE age = ?'; SET @age = 25; EXECUTE stmt USING @age;
Benefits of Using Query Parameters
Security
- Prevents SQL injection, as the values are bound to placeholders, not concatenated directly into the query string.
Performance
- Improves execution speed for repeated queries, as the database server parses and compiles the query only once.
Flexibility
- Allows for dynamic queries without the need for string concatenation.
How to Use Query Parameters
Creating a Prepared Statement
Use PREPARE
to create a prepared statement with placeholders.
PREPARE stmt FROM 'INSERT INTO products (name, price) VALUES (?, ?)';
Binding Parameters
Bind values to the placeholders using SET
.
SET @productName = 'Laptop', @productPrice = 1000;
Executing the Statement
Execute the prepared statement using EXECUTE
with the bound parameters.
EXECUTE stmt USING @productName, @productPrice;
Deallocating the Prepared Statement
Release the prepared statement after use with DEALLOCATE PREPARE
.
DEALLOCATE PREPARE stmt;
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

Common Use Cases
Dynamic Filtering in SELECT Queries
Used for filtering results based on variable criteria.
PREPARE stmt FROM 'SELECT * FROM employees WHERE department = ?';
Inserting User-Generated Data
Safely insert data provided by users, such as in web forms.
PREPARE stmt FROM 'INSERT INTO feedback (user_id, comment) VALUES (?, ?)';
Updating Records with Variable Data
Update records where the values are not known beforehand.
PREPARE stmt FROM 'UPDATE accounts SET balance = balance - ? WHERE account_id = ?';
Deleting Records Based on Conditions
Delete records dynamically based on certain conditions.
PREPARE stmt FROM 'DELETE FROM logs WHERE created_at < ?';
Best Practices
- Always use query parameters instead of string concatenation for user input.
- Release prepared statements when they are no longer needed.
- Regularly review and optimize your prepared statements.
Further Reading
For those looking to further optimize their database interactions and manage their SQL queries more effectively, Basedash offers tools to create and share SQL queries, manage permissions, and build data dashboards, all with a focus on simplicity and collaboration.
TOC
January 4, 2024
MySQL query parameters are placeholders in SQL statements that are replaced with actual values during execution. They enhance security by preventing SQL injection attacks and improve query efficiency by allowing the database to cache prepared statements.
Understanding Query Parameters
Query parameters in MySQL are used in prepared statements. A prepared statement is a feature used to execute the same statement repeatedly with high efficiency. Parameters in these statements act as placeholders for actual values that are substituted in at execution time.
Example of a Query Parameter
PREPARE stmt FROM 'SELECT * FROM users WHERE age = ?'; SET @age = 25; EXECUTE stmt USING @age;
Benefits of Using Query Parameters
Security
- Prevents SQL injection, as the values are bound to placeholders, not concatenated directly into the query string.
Performance
- Improves execution speed for repeated queries, as the database server parses and compiles the query only once.
Flexibility
- Allows for dynamic queries without the need for string concatenation.
How to Use Query Parameters
Creating a Prepared Statement
Use PREPARE
to create a prepared statement with placeholders.
PREPARE stmt FROM 'INSERT INTO products (name, price) VALUES (?, ?)';
Binding Parameters
Bind values to the placeholders using SET
.
SET @productName = 'Laptop', @productPrice = 1000;
Executing the Statement
Execute the prepared statement using EXECUTE
with the bound parameters.
EXECUTE stmt USING @productName, @productPrice;
Deallocating the Prepared Statement
Release the prepared statement after use with DEALLOCATE PREPARE
.
DEALLOCATE PREPARE stmt;
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

Common Use Cases
Dynamic Filtering in SELECT Queries
Used for filtering results based on variable criteria.
PREPARE stmt FROM 'SELECT * FROM employees WHERE department = ?';
Inserting User-Generated Data
Safely insert data provided by users, such as in web forms.
PREPARE stmt FROM 'INSERT INTO feedback (user_id, comment) VALUES (?, ?)';
Updating Records with Variable Data
Update records where the values are not known beforehand.
PREPARE stmt FROM 'UPDATE accounts SET balance = balance - ? WHERE account_id = ?';
Deleting Records Based on Conditions
Delete records dynamically based on certain conditions.
PREPARE stmt FROM 'DELETE FROM logs WHERE created_at < ?';
Best Practices
- Always use query parameters instead of string concatenation for user input.
- Release prepared statements when they are no longer needed.
- Regularly review and optimize your prepared statements.
Further Reading
For those looking to further optimize their database interactions and manage their SQL queries more effectively, Basedash offers tools to create and share SQL queries, manage permissions, and build data dashboards, all with a focus on simplicity and collaboration.
January 4, 2024
MySQL query parameters are placeholders in SQL statements that are replaced with actual values during execution. They enhance security by preventing SQL injection attacks and improve query efficiency by allowing the database to cache prepared statements.
Understanding Query Parameters
Query parameters in MySQL are used in prepared statements. A prepared statement is a feature used to execute the same statement repeatedly with high efficiency. Parameters in these statements act as placeholders for actual values that are substituted in at execution time.
Example of a Query Parameter
PREPARE stmt FROM 'SELECT * FROM users WHERE age = ?'; SET @age = 25; EXECUTE stmt USING @age;
Benefits of Using Query Parameters
Security
- Prevents SQL injection, as the values are bound to placeholders, not concatenated directly into the query string.
Performance
- Improves execution speed for repeated queries, as the database server parses and compiles the query only once.
Flexibility
- Allows for dynamic queries without the need for string concatenation.
How to Use Query Parameters
Creating a Prepared Statement
Use PREPARE
to create a prepared statement with placeholders.
PREPARE stmt FROM 'INSERT INTO products (name, price) VALUES (?, ?)';
Binding Parameters
Bind values to the placeholders using SET
.
SET @productName = 'Laptop', @productPrice = 1000;
Executing the Statement
Execute the prepared statement using EXECUTE
with the bound parameters.
EXECUTE stmt USING @productName, @productPrice;
Deallocating the Prepared Statement
Release the prepared statement after use with DEALLOCATE PREPARE
.
DEALLOCATE PREPARE stmt;
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

Common Use Cases
Dynamic Filtering in SELECT Queries
Used for filtering results based on variable criteria.
PREPARE stmt FROM 'SELECT * FROM employees WHERE department = ?';
Inserting User-Generated Data
Safely insert data provided by users, such as in web forms.
PREPARE stmt FROM 'INSERT INTO feedback (user_id, comment) VALUES (?, ?)';
Updating Records with Variable Data
Update records where the values are not known beforehand.
PREPARE stmt FROM 'UPDATE accounts SET balance = balance - ? WHERE account_id = ?';
Deleting Records Based on Conditions
Delete records dynamically based on certain conditions.
PREPARE stmt FROM 'DELETE FROM logs WHERE created_at < ?';
Best Practices
- Always use query parameters instead of string concatenation for user input.
- Release prepared statements when they are no longer needed.
- Regularly review and optimize your prepared statements.
Further Reading
For those looking to further optimize their database interactions and manage their SQL queries more effectively, Basedash offers tools to create and share SQL queries, manage permissions, and build data dashboards, all with a focus on simplicity and collaboration.
What is Basedash?
What is Basedash?
What is Basedash?
Basedash is the best MySQL admin panel
Basedash is the best MySQL admin panel
Basedash is the best MySQL admin panel
If you're building with MySQL, you need Basedash. It gives you an instantly generated admin panel to understand, query, build dashboards, edit, and share access to your data.
If you're building with MySQL, you need Basedash. It gives you an instantly generated admin panel to understand, query, build dashboards, edit, and share access to your data.
If you're building with MySQL, you need Basedash. It gives you an instantly generated admin panel to understand, query, build dashboards, edit, and share access to your data.




Dashboards and charts
Edit data, create records, oversee how your product is running without the need to build or manage custom software.
USER CRM
ADMIN PANEL
SQL COMPOSER WITH AI

Related posts
Related posts
Related posts



How to Add Columns to MySQL Tables with ALTER TABLE
Robert Cooper



How to Add Columns to Your MySQL Table
Max Musing



Pivot Tables in MySQL
Robert Cooper



How to Rename a Table in MySQL
Max Musing
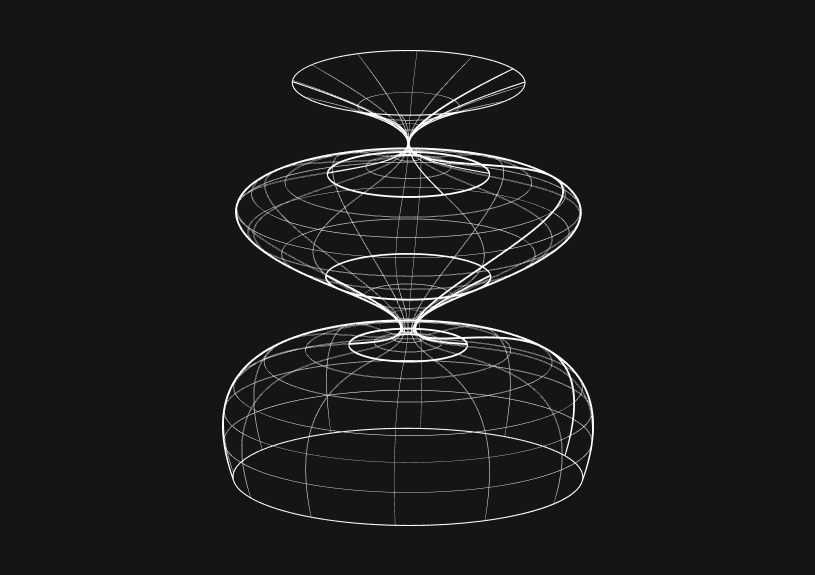
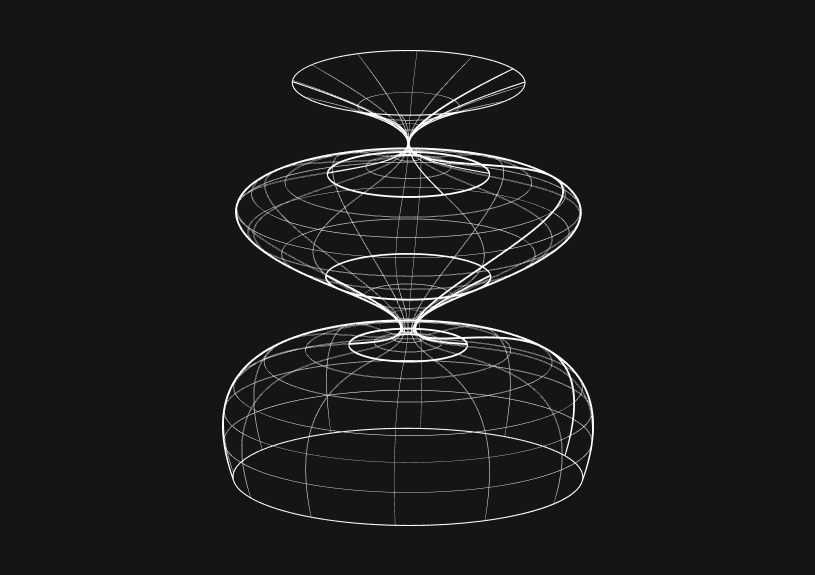
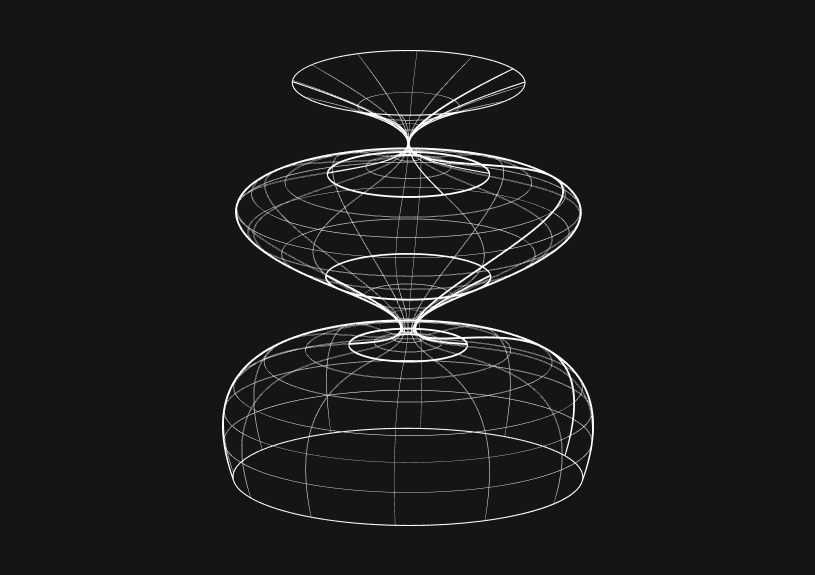
How to Optimize MySQL Tables for Better Performance
Robert Cooper



How to Display MySQL Table Schema: A Guide
Jeremy Sarchet