
MySQL Select Random 10 Rows
January 5, 2024
Selecting random rows from a database is a common task in SQL, particularly useful for sampling data or generating random subsets for analysis. In MySQL, this can be achieved using various methods, each with its own set of advantages.
Understanding the RAND() Function
The simplest way to select random rows is by using the RAND()
function. This function generates a random floating-point value between 0 and 1 for each row, allowing you to sort and limit your selection.
Basic Random Selection
SELECT * FROM your_table ORDER BY RAND() LIMIT 10;
This query selects 10 random rows from your_table
. The ORDER BY RAND()
clause randomizes the row order, and the LIMIT 10
clause restricts the output to 10 rows.
Considerations
- Performance: This method is straightforward but can be slow for large tables because
RAND()
is evaluated for every row. - Reproducibility: The randomness means you might get different results each time you run the query.
Using Primary Key for Random Selection
If your table has a numeric primary key with relatively few gaps, you can use it for more efficient random selection.
Random Selection with Primary Key
SELECT * FROM your_table WHERE primary_key_column >= (SELECT FLOOR(MAX(primary_key_column) * RAND()) FROM your_table) ORDER BY primary_key_column LIMIT 10;
This query randomly selects a starting point based on the primary key and retrieves the next 10 rows.
Considerations
- Efficiency: This method is typically faster than using
RAND()
on the entire table. - Distribution: The randomness can be skewed if the primary keys are not evenly distributed.
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

Random Sampling with User Variables
For more control over randomness, especially in large datasets, you can use user variables to assign a random number to each row and then select based on these numbers.
Random Sampling Query
SET @row_number = 0; SELECT * FROM ( SELECT *, (@row_number:=@row_number + 1) AS num FROM your_table ORDER BY RAND() ) AS t WHERE num % (SELECT ROUND(COUNT(*) / 10) FROM your_table) = 0 LIMIT 10;
This query first assigns a row number to each row in a random order, then selects rows based on these numbers.
Considerations
- Customization: This method allows for more complex selection criteria.
- Overhead: The additional complexity might introduce more computational overhead.
Conclusion
Choosing the right method for selecting random rows in MySQL depends on your specific requirements, such as the size of your dataset and the need for reproducibility or performance. Experiment with different approaches to find the most suitable one for your scenario.
TOC
January 5, 2024
Selecting random rows from a database is a common task in SQL, particularly useful for sampling data or generating random subsets for analysis. In MySQL, this can be achieved using various methods, each with its own set of advantages.
Understanding the RAND() Function
The simplest way to select random rows is by using the RAND()
function. This function generates a random floating-point value between 0 and 1 for each row, allowing you to sort and limit your selection.
Basic Random Selection
SELECT * FROM your_table ORDER BY RAND() LIMIT 10;
This query selects 10 random rows from your_table
. The ORDER BY RAND()
clause randomizes the row order, and the LIMIT 10
clause restricts the output to 10 rows.
Considerations
- Performance: This method is straightforward but can be slow for large tables because
RAND()
is evaluated for every row. - Reproducibility: The randomness means you might get different results each time you run the query.
Using Primary Key for Random Selection
If your table has a numeric primary key with relatively few gaps, you can use it for more efficient random selection.
Random Selection with Primary Key
SELECT * FROM your_table WHERE primary_key_column >= (SELECT FLOOR(MAX(primary_key_column) * RAND()) FROM your_table) ORDER BY primary_key_column LIMIT 10;
This query randomly selects a starting point based on the primary key and retrieves the next 10 rows.
Considerations
- Efficiency: This method is typically faster than using
RAND()
on the entire table. - Distribution: The randomness can be skewed if the primary keys are not evenly distributed.
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

Random Sampling with User Variables
For more control over randomness, especially in large datasets, you can use user variables to assign a random number to each row and then select based on these numbers.
Random Sampling Query
SET @row_number = 0; SELECT * FROM ( SELECT *, (@row_number:=@row_number + 1) AS num FROM your_table ORDER BY RAND() ) AS t WHERE num % (SELECT ROUND(COUNT(*) / 10) FROM your_table) = 0 LIMIT 10;
This query first assigns a row number to each row in a random order, then selects rows based on these numbers.
Considerations
- Customization: This method allows for more complex selection criteria.
- Overhead: The additional complexity might introduce more computational overhead.
Conclusion
Choosing the right method for selecting random rows in MySQL depends on your specific requirements, such as the size of your dataset and the need for reproducibility or performance. Experiment with different approaches to find the most suitable one for your scenario.
January 5, 2024
Selecting random rows from a database is a common task in SQL, particularly useful for sampling data or generating random subsets for analysis. In MySQL, this can be achieved using various methods, each with its own set of advantages.
Understanding the RAND() Function
The simplest way to select random rows is by using the RAND()
function. This function generates a random floating-point value between 0 and 1 for each row, allowing you to sort and limit your selection.
Basic Random Selection
SELECT * FROM your_table ORDER BY RAND() LIMIT 10;
This query selects 10 random rows from your_table
. The ORDER BY RAND()
clause randomizes the row order, and the LIMIT 10
clause restricts the output to 10 rows.
Considerations
- Performance: This method is straightforward but can be slow for large tables because
RAND()
is evaluated for every row. - Reproducibility: The randomness means you might get different results each time you run the query.
Using Primary Key for Random Selection
If your table has a numeric primary key with relatively few gaps, you can use it for more efficient random selection.
Random Selection with Primary Key
SELECT * FROM your_table WHERE primary_key_column >= (SELECT FLOOR(MAX(primary_key_column) * RAND()) FROM your_table) ORDER BY primary_key_column LIMIT 10;
This query randomly selects a starting point based on the primary key and retrieves the next 10 rows.
Considerations
- Efficiency: This method is typically faster than using
RAND()
on the entire table. - Distribution: The randomness can be skewed if the primary keys are not evenly distributed.
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

Random Sampling with User Variables
For more control over randomness, especially in large datasets, you can use user variables to assign a random number to each row and then select based on these numbers.
Random Sampling Query
SET @row_number = 0; SELECT * FROM ( SELECT *, (@row_number:=@row_number + 1) AS num FROM your_table ORDER BY RAND() ) AS t WHERE num % (SELECT ROUND(COUNT(*) / 10) FROM your_table) = 0 LIMIT 10;
This query first assigns a row number to each row in a random order, then selects rows based on these numbers.
Considerations
- Customization: This method allows for more complex selection criteria.
- Overhead: The additional complexity might introduce more computational overhead.
Conclusion
Choosing the right method for selecting random rows in MySQL depends on your specific requirements, such as the size of your dataset and the need for reproducibility or performance. Experiment with different approaches to find the most suitable one for your scenario.
What is Basedash?
What is Basedash?
What is Basedash?
Basedash is the best MySQL admin panel
Basedash is the best MySQL admin panel
Basedash is the best MySQL admin panel
If you're building with MySQL, you need Basedash. It gives you an instantly generated admin panel to understand, query, build dashboards, edit, and share access to your data.
If you're building with MySQL, you need Basedash. It gives you an instantly generated admin panel to understand, query, build dashboards, edit, and share access to your data.
If you're building with MySQL, you need Basedash. It gives you an instantly generated admin panel to understand, query, build dashboards, edit, and share access to your data.




Dashboards and charts
Edit data, create records, oversee how your product is running without the need to build or manage custom software.
USER CRM
ADMIN PANEL
SQL COMPOSER WITH AI

Related posts
Related posts
Related posts



How to Add Columns to MySQL Tables with ALTER TABLE
Robert Cooper



How to Add Columns to Your MySQL Table
Max Musing



Pivot Tables in MySQL
Robert Cooper



How to Rename a Table in MySQL
Max Musing
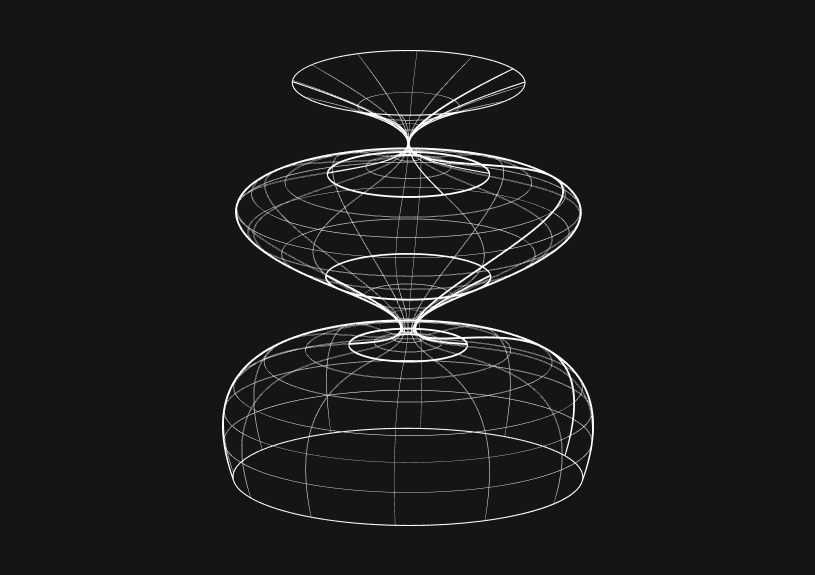
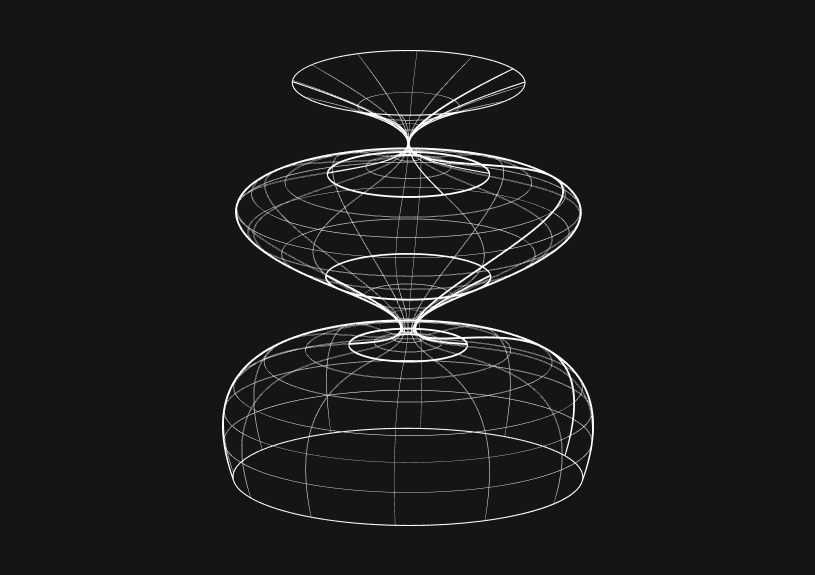
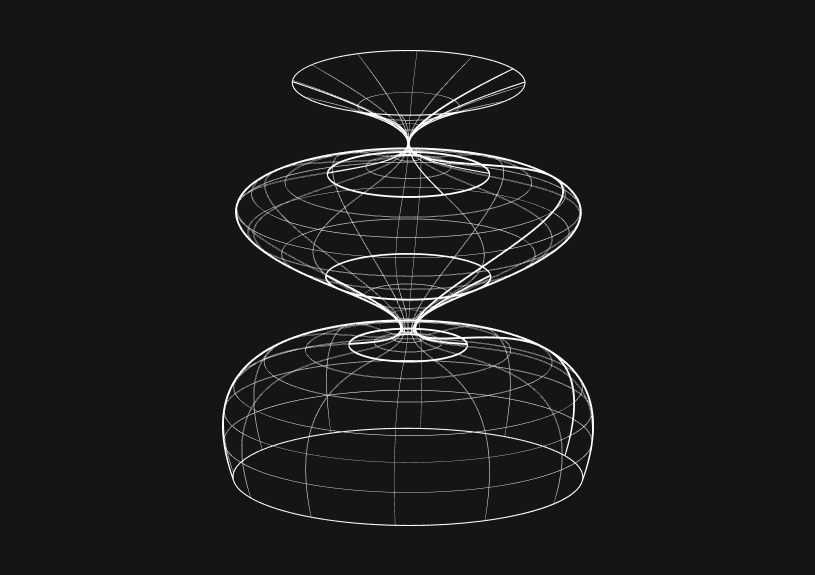
How to Optimize MySQL Tables for Better Performance
Robert Cooper



How to Display MySQL Table Schema: A Guide
Jeremy Sarchet